Accelerating Whisper Inference with Speculative Decoding: Doubling Speed Without Sacrificing Accuracy
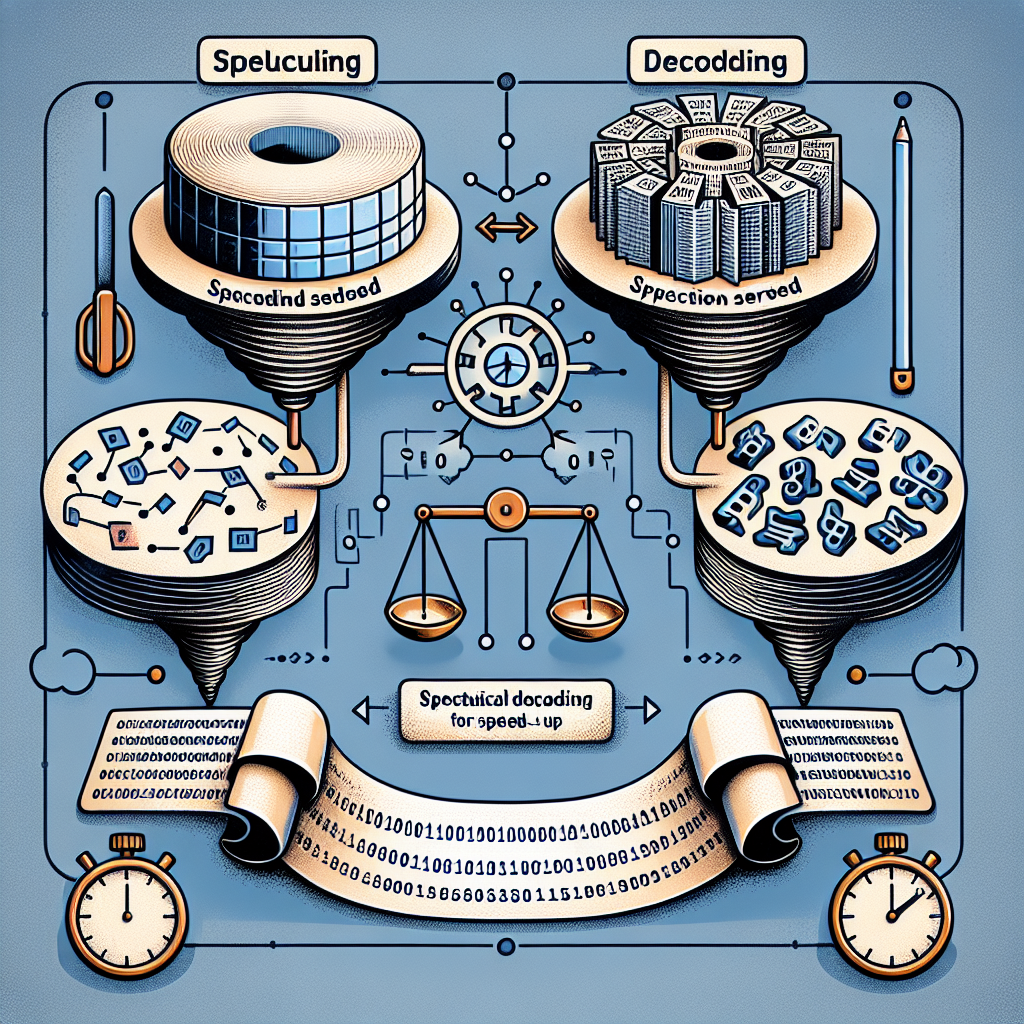
Introduction
In the realm of speech-to-text technology, the quest for efficiency and accuracy is perpetual. Among the notable advancements, OpenAI's Whisper model has emerged as a paragon of excellence, setting new benchmarks in the transcription of spoken language into written text. This general-purpose speech transcription model has not only demonstrated remarkable accuracy across a diverse array of benchmarks and audio conditions but has also shown proficiency in understanding and transcribing multilingual audio inputs.
The Whisper Model: A Benchmark of Excellence
Whisper's latest iteration, the large-v3 model, has clinched the top position on the OpenASR Leaderboard, earning accolades as the premier open-source speech transcription solution for English. Its prowess extends beyond English, achieving a word error rate (WER) of less than 30% across an impressive 42 out of 58 languages tested in the Common Voice 15 dataset. This multilingual capability positions Whisper as a versatile tool in global communication, breaking down language barriers and facilitating clearer understanding.
The Challenge of Inference Time
Despite its transcriptional accuracy, Whisper's Achilles' heel lies in its inference speed. Transcribing a one-hour audio clip can take upwards of six minutes on a 16GB T4 GPU, even after applying inference optimizations such as flash attention, half-precision, and chunking. This bottleneck in processing speed poses challenges, especially in real-time applications or scenarios demanding quick turnaround times.
Introducing Speculative Decoding
To address this challenge, we introduce Speculative Decoding—a groundbreaking method that propels Whisper's inference time to unprecedented speeds. By harnessing this technique, we can halve the inference duration without compromising the model's accuracy. This innovative approach provides a seamless upgrade to existing Whisper pipelines, offering a substantial speed boost while maintaining the high-quality transcription output that users have come to expect.
Speculative Decoding operates on a simple yet powerful premise: by employing a faster, assistant model to generate candidate tokens, and then verifying these tokens with the main model, we can significantly accelerate the transcription process. This method not only quickens the pace of transcription but also ensures that the final output remains true to the accuracy standards set by the main Whisper model.
The Perfect Balance Between Speed and Accuracy
This introduction to Speculative Decoding sets the stage for a deeper exploration of its mechanisms, implementations, and practical applications. As we delve further into this topic, we will uncover how this method strikes an optimal balance between speed and accuracy, thereby enhancing the utility and applicability of the Whisper model in diverse contexts. Join us as we journey through the intricacies of Speculative Decoding and its transformative impact on speech transcription technology.
Overview
In the realm of speech transcription, OpenAI's Whisper has set a new benchmark, establishing itself as a front-runner across various performance metrics and linguistic environments. With its latest iteration, the large-v3 model, it has ascended to the pinnacle of the OpenASR Leaderboard, heralded as the premier open-source solution for English speech transcription. Its prowess extends beyond the English lexicon, demonstrating commendable multilingual capabilities by securing a word error rate (WER) of under 30% in 42 out of 58 languages assessed within the Common Voice 15 dataset.
Despite its impressive transcription accuracy, Whisper's Achilles' heel lies in its inference speed. The transcription of a one-hour audio clip could extend beyond six minutes on a 16GB T4 GPU, even after the application of inference optimizations such as flash attention, half-precision computation, and chunking strategies.
Enter Speculative Decoding - a groundbreaking methodology aimed at halving Whisper's inference duration without compromising the quality of its output. This technique is a marvel of innovation, guaranteeing identical results from the model by mathematical assurance. It emerges as an ideal substitute for existing Whisper workflows, promising a seamless 2x speed enhancement while preserving accuracy integrity. For an abridged exposition of this blog post, complete with code yet concise in explanations, an accompanying Google Colab is available for consultation.
Speculative Decoding Explored
Conceived by Yaniv Leviathan and colleagues at Google, Speculative Decoding introduces a paradigm where a nimble, assistant model predicts a sequence of candidate tokens which are subsequently validated by the larger, primary model. This synergy not only accelerates the decoding process but also ensures fidelity to the original model's output, making it a flawless integration into existing Whisper pipelines.
English Speech Transcription Reimagined
Our baseline evaluation of Whisper large-v2 lays the groundwork, setting the stage for a transformative comparison with Speculative Decoding in action. By employing an assistant model significantly faster than the main one, we navigate the trade-off between speed and accuracy, leaning towards rapidity due to the preponderance of "easier" tokens in typical datasets.
Multilingual Speech Transcription Enhanced
The versatility of Speculative Decoding extends to multilingual transcription, necessitating an assistant model compatible with the main model's vocabulary. This section delves into the intricacies of selecting an appropriate assistant model for different variants of Whisper, ensuring a harmonious relationship that maximizes efficiency without sacrificing linguistic diversity.
Strategies for Efficient Speculative Decoding
This segment presents two pivotal strategies for optimizing Speculative Decoding: choosing an assistant model that balances speed with accuracy and fine-tuning both models to align their token distributions closely. It underscores the importance of model compatibility and shared vocabularies, providing a roadmap for implementing Speculative Decoding across various languages and Whisper versions.
In conclusion, Speculative Decoding stands as a beacon of innovation in the field of speech transcription, offering a dual boon of enhanced speed and unaltered accuracy. This overview has sketched the contours of this exciting development, inviting readers to explore the deeper technicalities and practical implementations that lie within the full blog post and its accompanying resources.
Utilizing Speculative Decoding in Python
Speculative Decoding is a groundbreaking technique designed to accelerate the inference process of machine learning models, notably those involved in speech transcription tasks. This method leverages a smaller, faster assistant model to predict a sequence of tokens which the main, more accurate model then verifies. The synergy between these two models yields a significantly faster inference time without compromising the quality of the output. Below, we delve into the practical steps required to implement this innovative approach using Python.
Setting Up Your Environment
Before embarking on the implementation journey, ensure your Python environment is properly set up with the necessary libraries. The foundation of this setup involves the Hugging Face transformers
and datasets
libraries, which facilitate the loading and processing of models and datasets, respectively.
pip install transformers datasets torch
Loading the Models
Main Model
The crux of Speculative Decoding hinges on the interaction between the main model and the assistant model. Begin by loading your main model, which offers the highest accuracy but at the cost of slower inference speeds. This model is responsible for the final verification of tokens predicted by the assistant model.
from transformers import AutoModelForSpeechSeq2Seq, AutoProcessor
model_id = "openai/whisper-large-v2"
device = "cuda" if torch.cuda.is_available() else "cpu"
main_model = AutoModelForSpeechSeq2Seq.from_pretrained(model_id).to(device)
processor = AutoProcessor.from_pretrained(model_id)
Assistant Model
Next, load the assistant model. This model is designed to be significantly faster than the main model, albeit less accurate. Its primary function is to quickly generate candidate tokens for verification by the main model.
assistant_model_id = "distil-whisper/distil-large-v2"
assistant_model = AutoModelForCausalLM.from_pretrained(assistant_model_id).to(device)
Implementing Speculative Decoding
With both models loaded, you can proceed to implement the speculative decoding process. This involves generating a sequence of candidate tokens with the assistant model and then verifying these tokens with the main model.
Generating Candidate Tokens
Use the assistant model to predict a sequence of tokens based on the input data. This step is crucial for speeding up the overall inference process.
def generate_candidate_tokens(assistant_model, inputs):
# Implement the logic to generate candidate tokens
pass
Verifying Tokens with the Main Model
Once you have a sequence of candidate tokens, pass them to the main model for verification. This ensures the accuracy of the final output while benefiting from the speed improvement offered by the assistant model.
def verify_tokens_with_main_model(main_model, candidate_tokens):
# Implement the logic to verify candidate tokens with the main model
pass
Optimizing the Process
To maximize the efficiency of speculative decoding, consider the following optimizations:
- Batch Processing: Process multiple input samples in a single batch to leverage GPU acceleration more effectively.
- Precision Tuning: Utilize mixed-precision computing (e.g., using float16 tensors) to further speed up the inference without a significant loss in accuracy.
- Token Distribution Alignment: Ensure the assistant model is trained in a way that its token distribution closely aligns with that of the main model to reduce the verification workload.
By meticulously implementing these steps and optimizations, you can significantly enhance the inference speed of your speech transcription models without sacrificing output quality. Speculative decoding thus emerges as a compelling technique for applications demanding both high accuracy and efficiency.
In this detailed exploration, we embarked on a journey to illuminate the innovative approach of speculative decoding, particularly within the ambit of the Whisper model for efficient speech transcription. Our foray into this domain revealed the potential to achieve significant enhancements in processing speed, effectively doubling the inference velocity, all while upholding the integrity and precision of the original outputs. This breakthrough holds substantial promise for those utilizing the Whisper model in their workflows, offering a seamless integration that retains the fidelity of transcription results without compromise.
Enhanced Efficiency with Speculative Decoding
The essence of speculative decoding lies in its ingenious utilization of a nimble assistant model, working in concert with the more robust main model to predict and verify token sequences. This partnership not only accelerates the transcription process but also ensures that the end results remain unchanged, offering a blend of speed and accuracy that is highly desirable in computational tasks. The implications of this are profound, offering users the ability to process audio files in nearly half the time previously required, without any degradation in the quality of the transcribed text.
Strategic Implementation for Maximized Performance
Assistant Model Selection
Choosing the right assistant model is pivotal for harnessing the full potential of speculative decoding. The goal is to identify a model that is significantly faster than the main model while maintaining a high degree of accuracy for the majority of token predictions. This strategic selection is crucial for optimizing performance and achieving the desired balance between speed and accuracy. By carefully selecting and potentially customizing the assistant model, users can tailor the speculative decoding process to fit their specific needs and maximize efficiency gains.
Batch Size Considerations
Another critical aspect to consider for optimizing speculative decoding performance is the batch size. It's been observed that the most substantial speed improvements are realized with a batch size of one. This is due to the mechanism of speculative decoding, where the alignment of candidate tokens across the batch plays a crucial role. Larger batch sizes may inadvertently slow down the process, as discrepancies in token validation across samples can lead to inefficiencies. Therefore, adhering to smaller batch sizes is recommended to fully leverage the speed advantages of speculative decoding.
Embracing Speculative Decoding in Your Workflow
The advent of speculative decoding as a methodological enhancement for the Whisper model represents a significant leap forward in speech transcription technology. By effectively doubling the inference speed without sacrificing accuracy, speculative decoding emerges as an invaluable tool for anyone seeking to optimize their transcription processes. We encourage practitioners and enthusiasts alike to consider integrating speculative decoding into their existing Whisper pipelines. The combination of minimal integration overhead, the assurance of maintained transcription quality, and significant performance gains makes speculative decoding an attractive proposition for enhancing the efficiency and effectiveness of speech transcription endeavors.
Final Thoughts
As we conclude this discourse on speculative decoding, it's clear that the benefits extend far beyond mere speed improvements. This technique stands as a testament to the power of innovative thinking in the realm of artificial intelligence and machine learning. By thoughtfully applying speculative decoding, we can unlock new levels of efficiency and performance in speech transcription, paving the way for more advanced applications and insights in the future.