Integrating Text-to-Speech in Python with Unreal Speech SDK: A Comprehensive Guide
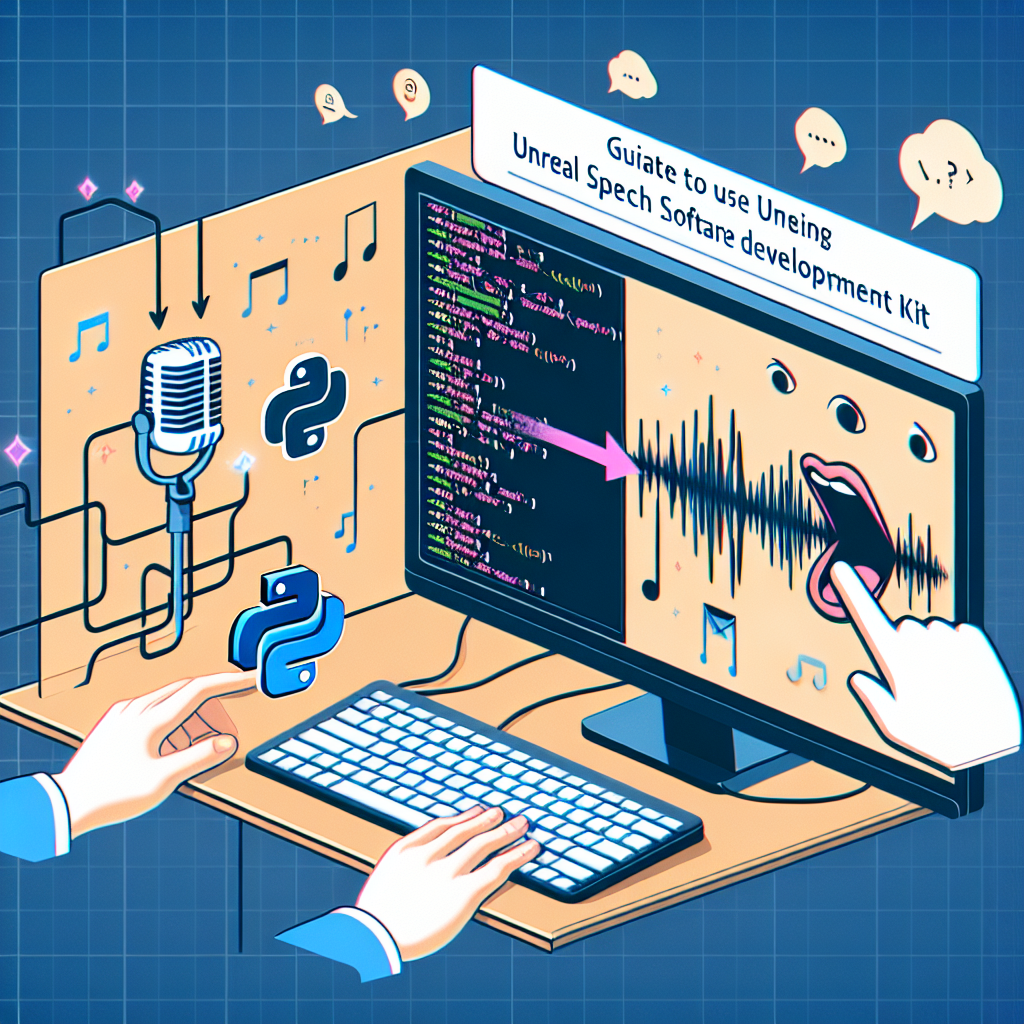
Introduction
Welcome to the comprehensive guide on integrating the Unreal Speech API into your Python projects. In this era of digital transformation, the ability to convert text into natural-sounding speech has become paramount for creating interactive applications, enhancing user experience, and making content more accessible. The Unreal Speech Python SDK serves as a bridge, allowing developers to harness the power of text-to-speech (TTS) synthesis with ease and efficiency.
Why Text-to-Speech?
In a world where multitasking has become the norm, TTS technology opens up new avenues for consuming content. From assisting visually impaired users to providing hands-free reading, the applications of TTS are vast and varied. Furthermore, it plays a crucial role in language learning, audiobook production, and even in the gaming industry, adding depth to storytelling.
Getting Started with Unreal Speech Python SDK
Embarking on your journey with the Unreal Speech Python SDK is a straightforward process designed for developers of all skill levels. This SDK not only simplifies the integration of TTS capabilities into your applications but also provides a robust set of features to tailor the speech output according to your needs. Whether you're looking to stream audio for immediate feedback or manage large synthesis tasks, the SDK has got you covered.
Features at a Glance
- Ease of Use: With minimal setup, you can start generating realistic speech in your applications.
- Flexibility: Customize voice, speed, pitch, and bitrate to fit the context of your application.
- Efficiency: Optimized for both short and long text synthesis, ensuring seamless user experiences.
Installation and Dependencies
Getting started with the Unreal Speech Python SDK is as simple as installing the package and its dependencies. This ensures that you have all the necessary tools to begin text-to-speech synthesis without a hitch. The SDK's compatibility with popular audio libraries further enhances its utility, making it a versatile choice for developers.
A World of Possibilities
By integrating the Unreal Speech Python SDK into your projects, you unlock a world of possibilities. Whether it's developing educational software that speaks to students, creating immersive games with dynamic narratives, or building accessibility features into your apps, the SDK provides the foundation you need to innovate and impress.
In the following sections, we will dive deeper into the capabilities of the Unreal Speech Python SDK, exploring its functionalities and showcasing how you can leverage its features to elevate your projects. Stay tuned for an exciting journey into the realm of text-to-speech synthesis, where creativity meets technology.
Getting Started with the Python SDK
Integrating text-to-speech (TTS) into your Python projects has never been easier, thanks to the Unreal Speech Python SDK. This toolkit provides a seamless interface to the Unreal Speech API, enabling you to bring voices to your applications. Whether you're creating an educational tool, an accessibility feature, or just adding some fun auditory feedback, this guide will walk you through the basic steps to get started.
Installation
First and foremost, you need to incorporate the Unreal Speech API package into your Python environment. This is effortlessly done using pip
, Python's package installer. Run the following command in your terminal or command prompt to download and install the package:
pip install unrealspeech
After installing the main package, certain dependencies are required to ensure everything runs smoothly. Execute the commands below to install these additional packages:
pip install playsound pydub simpleaudio
These dependencies are crucial for audio playback and manipulation within your projects.
Initializing the UnrealSpeechAPI
To begin using the SDK, you must first import the UnrealSpeechAPI
class from the unrealspeech
package. Additionally, utility functions such as play
and save
are available for audio playback and saving. Here's how you do it:
from unrealspeech import UnrealSpeechAPI, play, save
Next, instantiate the UnrealSpeechAPI
class with your unique API key. This key connects your application to the Unreal Speech services, allowing you to access its features:
api_key = 'YOUR_API_KEY'
speech_api = UnrealSpeechAPI(api_key)
Make sure to replace 'YOUR_API_KEY'
with your actual API key obtained from Unreal Speech.
Generating and Playing Speech
Creating speech from text is straightforward with the Unreal Speech Python SDK. You can customize the voice, speed, pitch, and bitrate to suit your application's needs. Here's a simple example:
text_to_speech = "Welcome to our application. Enjoy your stay."
voice_id = "Scarlett" # Choose a voice that fits your audience
bitrate = "192k" # Higher bitrate for better quality
speed = 0 # Normal speed
pitch = 1.0 # Standard pitch
# Generating speech
audio_data = speech_api.speech(
text=text_to_speech,
voice_id=voice_id,
bitrate=bitrate,
speed=speed,
pitch=pitch
)
# Playing the generated speech
play(audio_data)
Streaming Audio for Immediate Playback
In scenarios where immediate feedback is crucial, such as alerts or notifications, you can stream audio directly. This bypasses the need to generate and then play the audio, providing a quicker response:
text_to_stream = "Alert: Your attention is required."
# Streaming audio
audio_data = speech_api.stream(
text=text_to_stream,
voice_id="Will",
bitrate="192k",
speed=0,
pitch=1.0
)
# Immediate playback
play(audio_data)
Managing Long Synthesis Tasks
For longer texts that require more processing time, the SDK offers an efficient way to manage synthesis tasks. This is especially useful for generating audiobooks, lengthy reports, or comprehensive guides:
# Creating a synthesis task for lengthy content
task_id = speech_api.create_synthesis_task(
text="Here is a long content example...",
voice_id="Dan",
bitrate="320k",
timestamp_type="word",
speed=0,
pitch=1.0
)
# Checking the status and retrieving the audio
audio_data = speech_api.get_synthesis_task_status(task_id)
# Playback
play(audio_data)
Saving Audio Files
Finally, saving the generated audio to a file allows for offline playback or embedding within other media. The SDK's save
function makes this process effortless:
# Generating speech to save
audio_data = speech_api.speech('Saving your audio is straightforward.')
# Saving to an MP3 file
save(audio_data, "saved_audio.mp3")
This enhanced guide aims to provide a comprehensive understanding of how to utilize the Unreal Speech Python SDK for text-to-speech synthesis. By following these steps, you can add dynamic and engaging auditory elements to your Python applications.