Making Automatic Speech Recognition on Large Files Feasible with Wav2Vec2 and Chunking Techniques
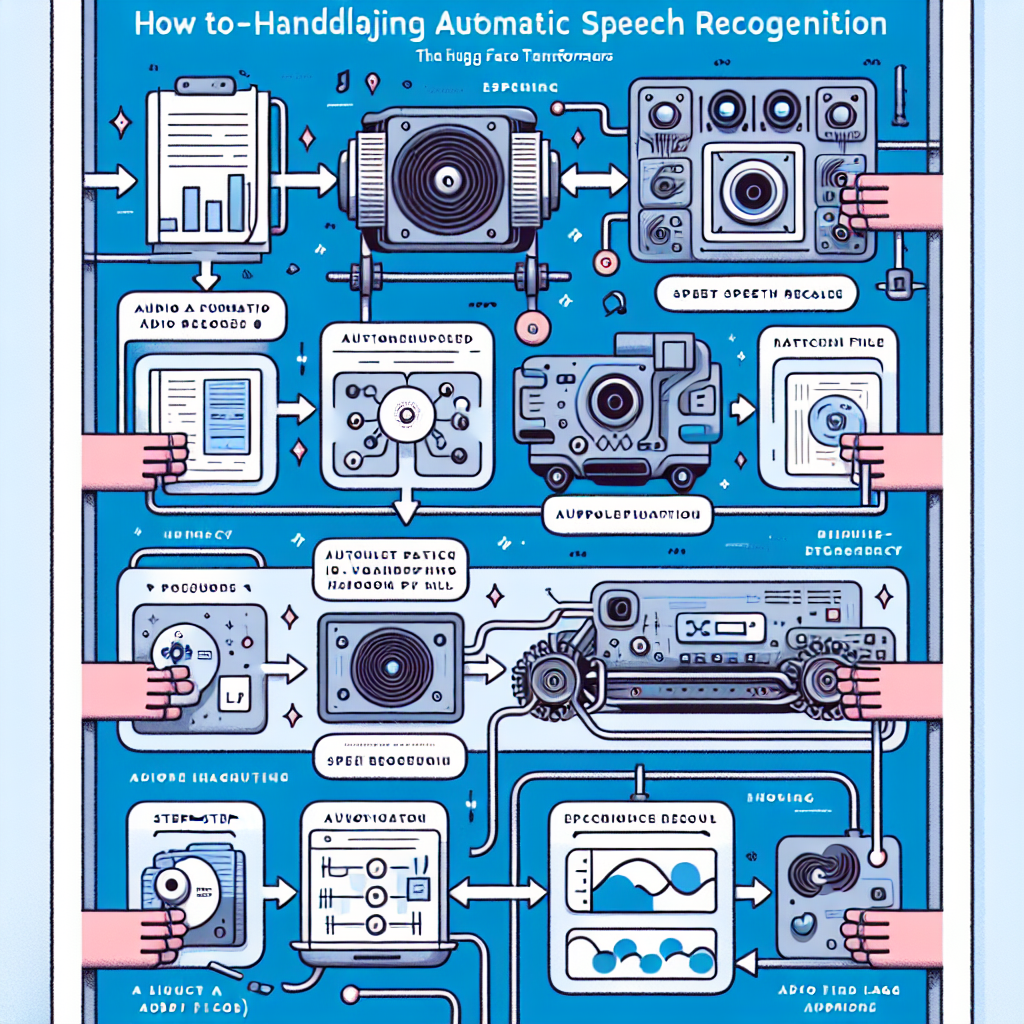
Introduction
In the rapidly evolving landscape of technology, automatic speech recognition (ASR) stands out as a groundbreaking advancement that has the potential to reshape how we interact with our devices. Among the plethora of models facilitating this transformation, Wav2Vec2, introduced by Meta AI Research in September 2020, has emerged as a frontrunner. This model, thanks to its innovative architecture, has significantly accelerated progress in self-supervised pre-training for speech recognition. Its popularity is evidenced by its impressive download statistics on the Hugging Face Hub, where it garners over a quarter of a million downloads monthly. However, one stumbling block that developers and researchers frequently encounter is the model's handling of lengthy audio files.
The Challenge with Large Files
Dealing with extensive audio files presents a unique set of challenges. At its core, Wav2Vec2 leverages transformer models, which, despite their numerous advantages, have a limitation in processing long sequences. This limitation stems not from the use of positional encodings, which Wav2Vec2 does not employ, but from the quadratic increase in computational complexity with respect to sequence length. Consequently, attempting to process an hour-long file, for instance, would overwhelm even the most robust GPUs, such as the NVIDIA A100, leading to inevitable crashes.
The Solution
Recognizing this challenge, the community has devised innovative strategies to make ASR feasible for files of any length or for live inference scenarios. These strategies revolve around the clever use of the Connectionist Temporal Classification (CTC) architecture that underpins Wav2Vec2. By exploiting the specific characteristics of CTC, we can achieve remarkably accurate speech recognition results, even with files that would traditionally be considered too long for processing.
Strategies for Overcoming the Limitation
Simple Chunking
The most straightforward approach involves dividing the lengthy audio files into smaller, more manageable chunks, such as segments of 10 seconds. This method, while computationally efficient, often results in suboptimal recognition quality, especially around the boundaries of the chunks.
Chunking with Stride
A more sophisticated strategy employs chunking with stride, allowing for overlapping chunks. This technique ensures that the model has adequate context in the center of each chunk, significantly improving the quality of speech recognition.
Enhancements for LM Augmented Models
Further refinements are possible with models augmented with a language model (LM), boosting word error rate (WER) performance without the need for fine-tuning. The integration of an LM directly with the logits allows for seamless application of the chunking with stride technique, enhancing the model's accuracy.
Live Inference
Leveraging the single-pass, fast-processing capability of CTC models like Wav2Vec2, live inference becomes a practical reality. By feeding the pipeline data in real-time and applying strategic striding, the model can deliver immediate transcription results, enhancing user experience in live scenarios.
This introduction aims to shed light on the transformative potential of Wav2Vec2 in the realm of automatic speech recognition. By addressing the challenges associated with processing lengthy audio files and live data streams, we unlock new possibilities for user interaction and accessibility. Through continuous innovation and strategic application of the model's capabilities, we can push the boundaries of what's possible in ASR technology, making it more versatile and effective than ever before.
Overview
The realm of Automatic Speech Recognition (ASR) has witnessed significant advancements, thanks to the advent of models like Wav2Vec2, developed by Meta AI Research. This model, since its introduction in September 2020, has revolutionized the approach to self-supervised pretraining for speech recognition. It has not only garnered attention for its innovative architecture but also for its impressive ability to understand and transcribe human speech with remarkable accuracy.
The Challenge with Large Audio Files
One of the inherent limitations when dealing with transformer-based models, such as Wav2Vec2, is their handling of long sequences. These models, despite their prowess, encounter constraints related to sequence length. This is not due to the use of positional encodings, as one might expect, but rather the quadratic cost associated with attention mechanisms. The computational demand skyrockets with an increase in sequence length, making it impractical to process hour-long audio files on standard hardware configurations.
Enter Chunking: A Simple Yet Effective Solution
To circumvent the limitations posed by large audio files, a straightforward method involves dividing the audio into manageable chunks. This process, commonly referred to as chunking, allows the model to perform inference on shorter segments of audio sequentially. While this approach offers computational efficiency, it traditionally sacrifices some degree of accuracy, particularly around the borders of these chunks where contextual information becomes crucial.
Stride-Based Chunking: Enhancing Contextual Understanding
Building upon the basic chunking methodology, the implementation of stride-based chunking presents a more refined solution. By allowing overlaps between chunks, the model is equipped with a broader context for each segment, thereby mitigating the accuracy drop-off at chunk borders. This technique leverages the Connectionist Temporal Classification (CTC) architecture inherent to Wav2Vec2, enabling the model to maintain high-quality speech recognition across the entirety of the audio file.
Expanding to Live Inference and LM Augmented Models
The versatility of Wav2Vec2 extends beyond static files, accommodating live inference scenarios and integration with Language Models (LM) for enhanced Word Error Rate (WER) performance. The stride-based chunking approach remains effective in these advanced applications, demonstrating the model's adaptability and the robustness of the underlying techniques.
In summary, the Wav2Vec2 model stands as a testament to the progress in ASR technology, offering innovative solutions to traditional challenges. Through strategic chunking methods and the effective use of CTC architecture, it achieves high-quality speech recognition, making it a valuable tool for a wide range of applications.
Utilizing Python for Enhanced Automatic Speech Recognition with Wav2Vec2
In the realm of automatic speech recognition (ASR), leveraging the power and flexibility of Python alongside the advanced capabilities of Wav2Vec2 models can significantly elevate the quality and efficiency of your ASR tasks. This guide aims to delve into the practical aspects of implementing Wav2Vec2 in Python for processing extensive audio files, ensuring you can handle even the most demanding ASR challenges with ease.
Setting Up Your Environment
Before diving into the coding aspect, it's crucial to establish a conducive development environment. This involves installing the necessary libraries, including the renowned transformers
library from Hugging Face, which houses the Wav2Vec2 model. Utilize the following command to ensure your Python environment is equipped with the latest version of this indispensable tool:
pip install transformers
Initializing the ASR Pipeline
The initial step in harnessing the Wav2Vec2 model for speech recognition involves setting up the ASR pipeline. This pipeline acts as a conduit, streamlining the flow of data from your audio files through the Wav2Vec2 model, ultimately producing transcribed text. The code snippet below illustrates how to initialize this pipeline using the transformers
library:
from transformers import pipeline
# Initialize the ASR pipeline with the Wav2Vec2 model
asr_pipeline = pipeline(model="facebook/wav2vec2-base-960h")
This line of code effectively creates an ASR pipeline utilizing the facebook/wav2vec2-base-960h
model, a pre-trained version of Wav2Vec2 known for its robust performance across a wide range of audio inputs.
Processing Large Audio Files
A common hurdle when working with ASR is the processing of large audio files. Due to hardware limitations and the inherent complexity of processing extensive audio sequences, directly feeding long audio files into the model can lead to suboptimal performance or even failure. To circumvent this, we employ a strategy of audio chunking with strides.
Basic Chunking Approach
The most straightforward method for handling large files is to divide the audio into smaller, manageable segments (chunks) and process each segment individually. This approach, while simple, ensures that the model can efficiently handle the input without being overwhelmed by its size. However, it's worth noting that this can sometimes lead to reduced accuracy around the boundaries of each chunk due to the lack of contextual information.
Advanced Chunking with Strides
To enhance the accuracy of ASR on large files, implementing chunking with strides offers a more sophisticated solution. This technique involves not only dividing the audio into chunks but also creating overlapping sections between these chunks. By doing so, each chunk retains a portion of the adjacent context, significantly improving the model's ability to accurately transcribe speech, especially at the boundaries of each chunk.
Here's how you can implement this advanced strategy in Python using the transformers
pipeline:
# Specify the length of each chunk and the stride lengths
chunk_length_s = 10 # in seconds
stride_length_s = (4, 2) # left and right strides in seconds
# Process a large audio file with chunking and strides
transcription = asr_pipeline("path/to/your/very_long_file.mp3", chunk_length_s=chunk_length_s, stride_length_s=stride_length_s)
This method ensures that you can process even very long audio files efficiently while maintaining high transcription accuracy. By adjusting the chunk length and stride parameters, you can fine-tune the balance between performance and accuracy to suit your specific needs.
This method ensures that you can process even very long audio files efficiently while maintaining high transcription accuracy. By adjusting the chunk length and stride parameters, you can fine-tune the balance between performance and accuracy to suit your specific needs.
Conclusion
By leveraging Python and the advanced features of Wav2Vec2 within the transformers
library, you can overcome the challenges associated with automatic speech recognition for large audio files. Through strategic chunking and the use of strides, it's possible to achieve high-quality transcriptions, ensuring that your ASR tasks are not only manageable but also remarkably accurate.
Conclusion
In this comprehensive exploration of harnessing Wav2Vec2 for automatic speech recognition (ASR) on extensive audio files, we've delved into the intricacies and innovative strategies that make processing large-scale audio data feasible and efficient. The utilization of Wav2Vec2 within the 🤗 Transformers framework showcases a significant leap towards overcoming the challenges associated with ASR, particularly when dealing with lengthy recordings or real-time inference scenarios.
Unveiling the Power of Chunking Strategies
We embarked on our journey by understanding the simple yet effective method of chunking, a technique that divides long audio files into manageable segments. This approach not only simplifies the ASR process but also optimizes computational resources. However, it's the introduction of stride-based chunking that truly revolutionizes our capability to maintain context and continuity in speech recognition. By strategically overlapping audio chunks, we ensure that the model has sufficient context around the borders, thereby enhancing the accuracy of transcriptions.
Enhancing ASR with Language Models
The augmentation of Wav2Vec2 with language models (LM) presents another layer of sophistication. This synergy between Wav2Vec2's robust framework and the nuanced understanding of language provided by LMs significantly boosts word error rate (WER) performance. It's a testament to the adaptability of the stride-based chunking method that it seamlessly integrates with LM-augmented models, further refining the quality of speech recognition without necessitating additional fine-tuning.
Pioneering Live Inference Capabilities
The exploration takes an exciting turn with the advent of live inference. Utilizing the single-pass, fast-processing nature of the CTC model inherent in Wav2Vec2, we pave the way for real-time speech transcription. This dynamic application of stride-based chunking to live audio feeds marks a pivotal advancement in making ASR more responsive and interactive. The potential for immediate transcription as speech occurs opens up new vistas for applications requiring instant feedback or interaction, from live captioning to interactive voice-controlled systems.
Through this detailed examination, we've not only highlighted the technical prowess of Wav2Vec2 and its compatibility with the 🤗 Transformers library but also illuminated the path forward for researchers, developers, and innovators seeking to push the boundaries of automatic speech recognition. The strategies and techniques discussed here offer a blueprint for tackling the inherent challenges of processing long audio files and live data streams, ensuring that the field of ASR continues to stride confidently into the future.
In summary, the journey through the capabilities of Wav2Vec2 within the context of large audio files and live inference has been enlightening. As we continue to explore and innovate within the realms of speech recognition, the insights gained from this exploration will undoubtedly serve as a cornerstone for future advancements in the field. Whether it's refining the chunking methodology or integrating more advanced language models, the quest for seamless, accurate, and efficient ASR is an ongoing endeavor that promises to reshape our interaction with technology.