Recreating AI-Generated Images with OpenAI GPT-4 and DALL-E 3 model
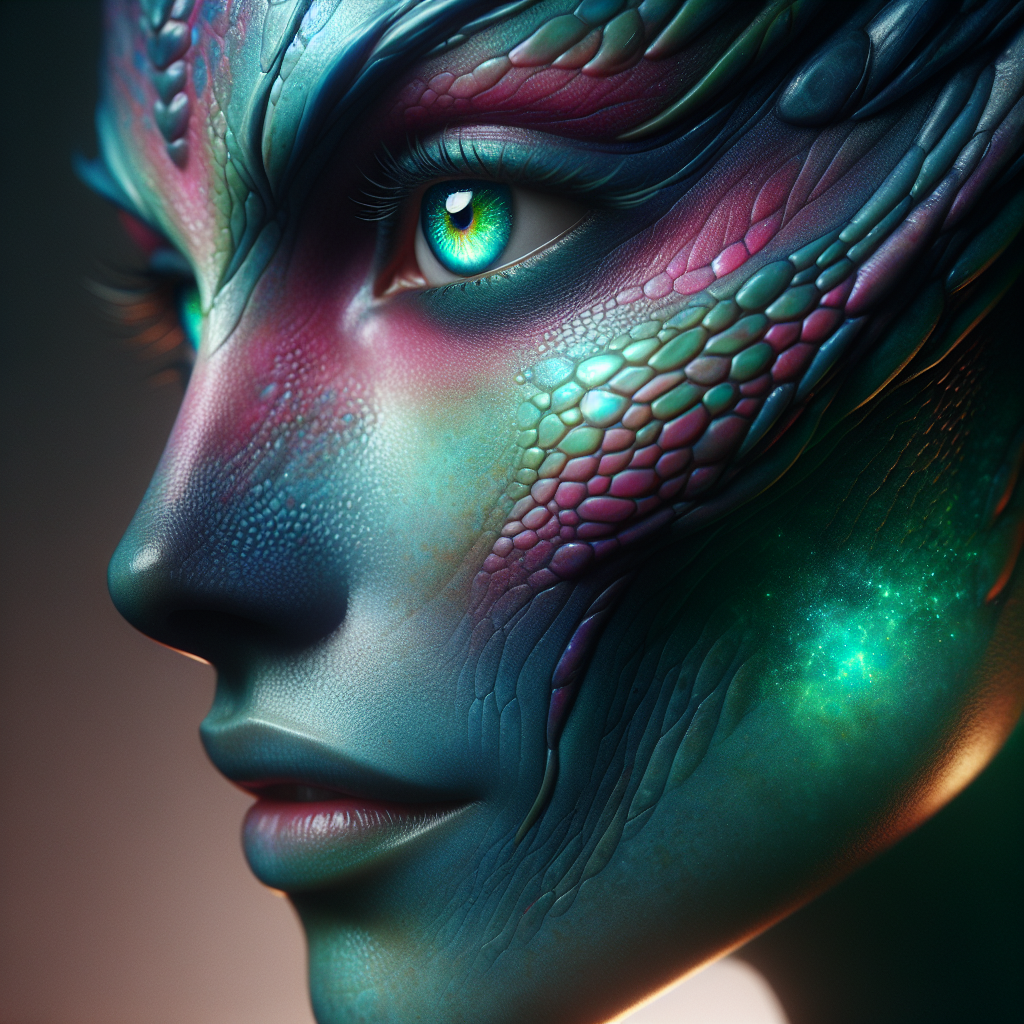
In the dynamic world of artificial intelligence, the ability to recreate images using advanced models like OpenAI's GPT-4 and DALL-E 3 opens up new frontiers for creativity and technology. This blog post delves into the process of recreating AI-generated images, providing a comprehensive guide complete with Python code.
Introduction to AI Image Recreation
The process of recreating images using AI involves two key components: image description and image generation. GPT-4, with its vision capabilities, is adept at describing images in detail. DALL-E 3, on the other hand, specializes in generating images from textual descriptions. By combining these two technologies, we can recreate an existing image or even create variations based on the original.
The Process Overview
- Image Description with GPT-4: Extract a detailed description of the image using GPT-4's vision capabilities.
- Image Generation with DALL-E 3: Use this description to generate a new image with DALL-E 3, which could be a recreation or a variation of the original.
Code Breakdown
The provided Python script is a succinct illustration of this process. Let's dissect it step-by-step for better understanding.
Setup and Dependencies
The script starts by importing necessary libraries such as os
, random
, string
, time
, requests
, and urllib
. It also imports openai
and dotenv
for API interactions and environment variable management.
import os
import random
import string
import time
import requests
from openai import OpenAI
from dotenv import load_dotenv
import urllib.request
load_dotenv()
loads environment variables, which typically include API keys for OpenAI.
The vision_api_describe_image
Function
This function takes an image URL as input and uses the GPT-4 vision model to generate a detailed description.
def vision_api_describe_image(url):
response = client.chat.completions.create(
model="gpt-4-vision-preview",
messages=[
{
"role": "user",
"content": [
{
"type": "image_url",
"image_url": {
"url": url
}
},
{
"type": "text",
"text": "Describe the image in detail (colors,lighting, camera, features, theme, style, etc)"
}
]
}
],
max_tokens=300
)
# accessing the content
description_text = response.choices[0].message.content
return description_text
The dalle_api_generate_image
Function
After obtaining the description, this function uses DALL-E 3 to generate an image based on that description.
def dalle_api_generate_image(description):
response = client.images.generate(
model="dall-e-3", prompt=description, size="1024x1024", quality="standard", n=1)
return response.data[0].url
Utility Function: generate_random_string
This helper function creates a random string, useful for generating unique filenames for the saved images.
def generate_random_string(length=6):
characters = string.ascii_letters + string.digits
random_string = ''.join(random.choice(characters) for _ in range(length))
return random_string
Main Execution Flow
In the main block, the script performs the following steps:
- Define a reference image URL.
- Get the description of the reference image using GPT-4.
- Use DALL-E 3 to generate images based on this description.
- Save the generated images locally.
if __name__ == "__main__":
reference_img = "https://cdn.pixabay.com/photo/2023/02/04/21/57/ai-generated-7768274_1280.jpg"
image_description = vision_api_describe_image(reference_img)
for _ in range(5):
synthetic_img = dalle_api_generate_image(image_description)
urllib.request.urlretrieve(
synthetic_img, f"images/{generate_random_string(6)}.jpg")
Reference image preview
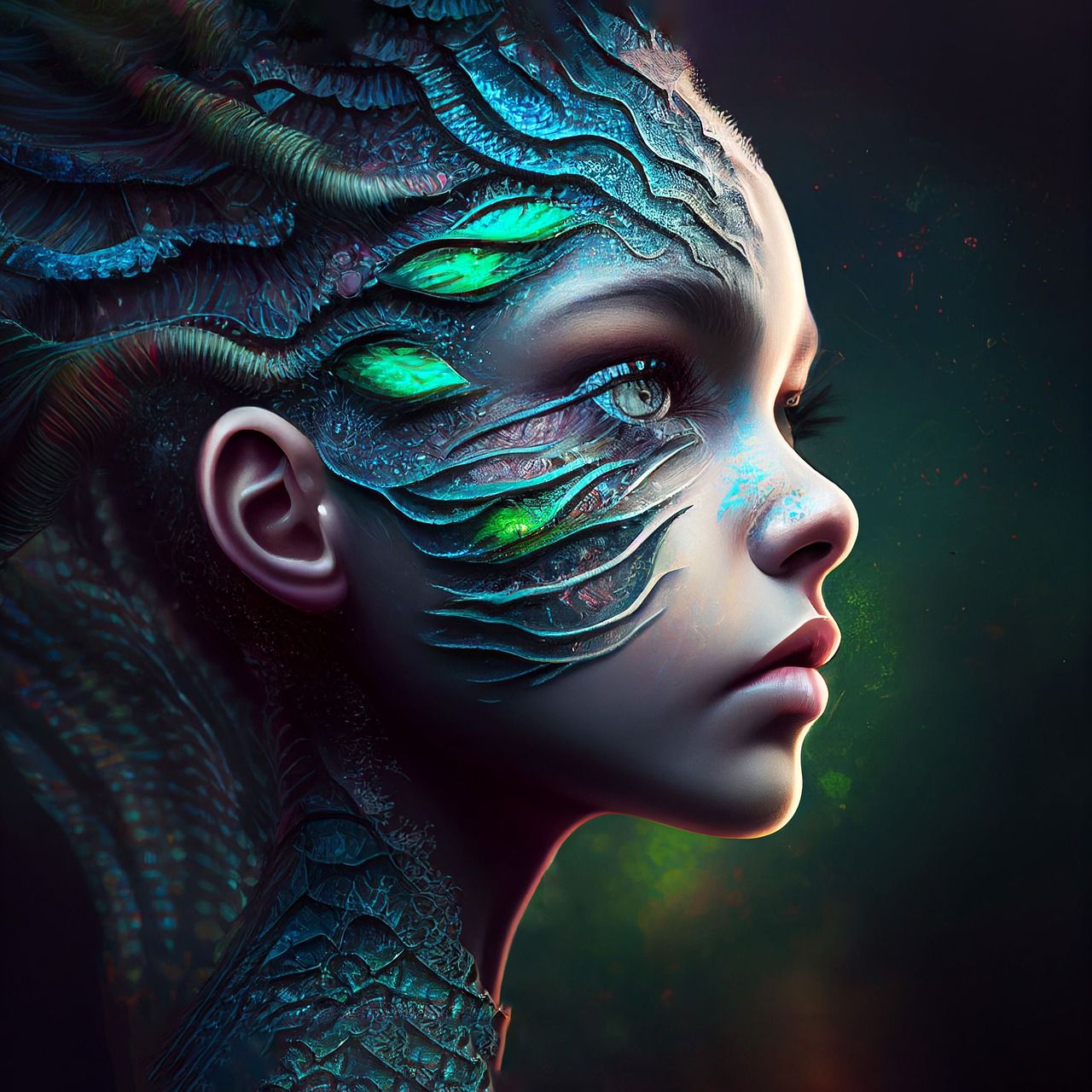
Practical Considerations
API Keys and Rate Limits
Ensure you have the necessary API keys and permissions from OpenAI. Be mindful of rate limits and usage costs associated with these APIs.
Handling Variability
The nature of AI means each generated image might slightly differ from the original, leading to unique and sometimes unexpected results.
Storage and Filenaming
The script saves images locally, which requires appropriate directory management and naming conventions to avoid overwrites and ensure easy retrieval.
Output of our script
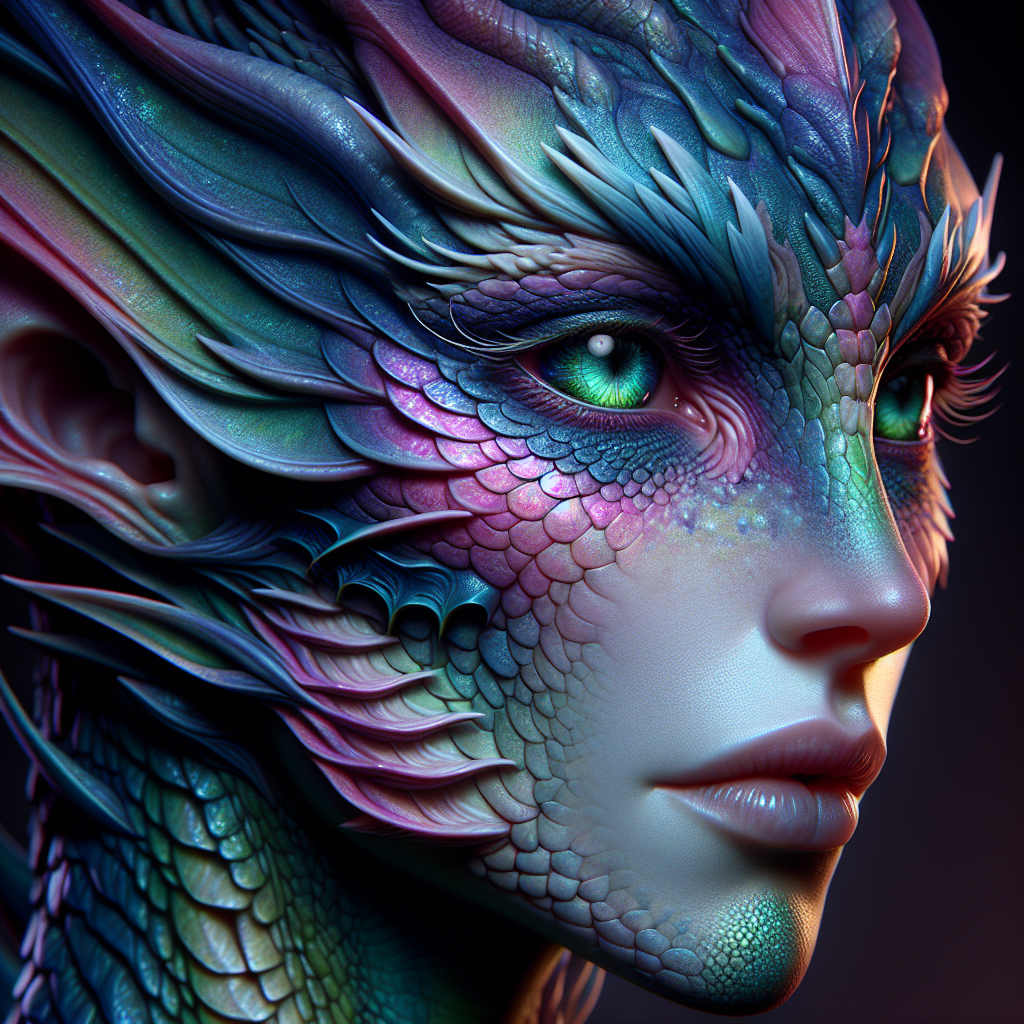
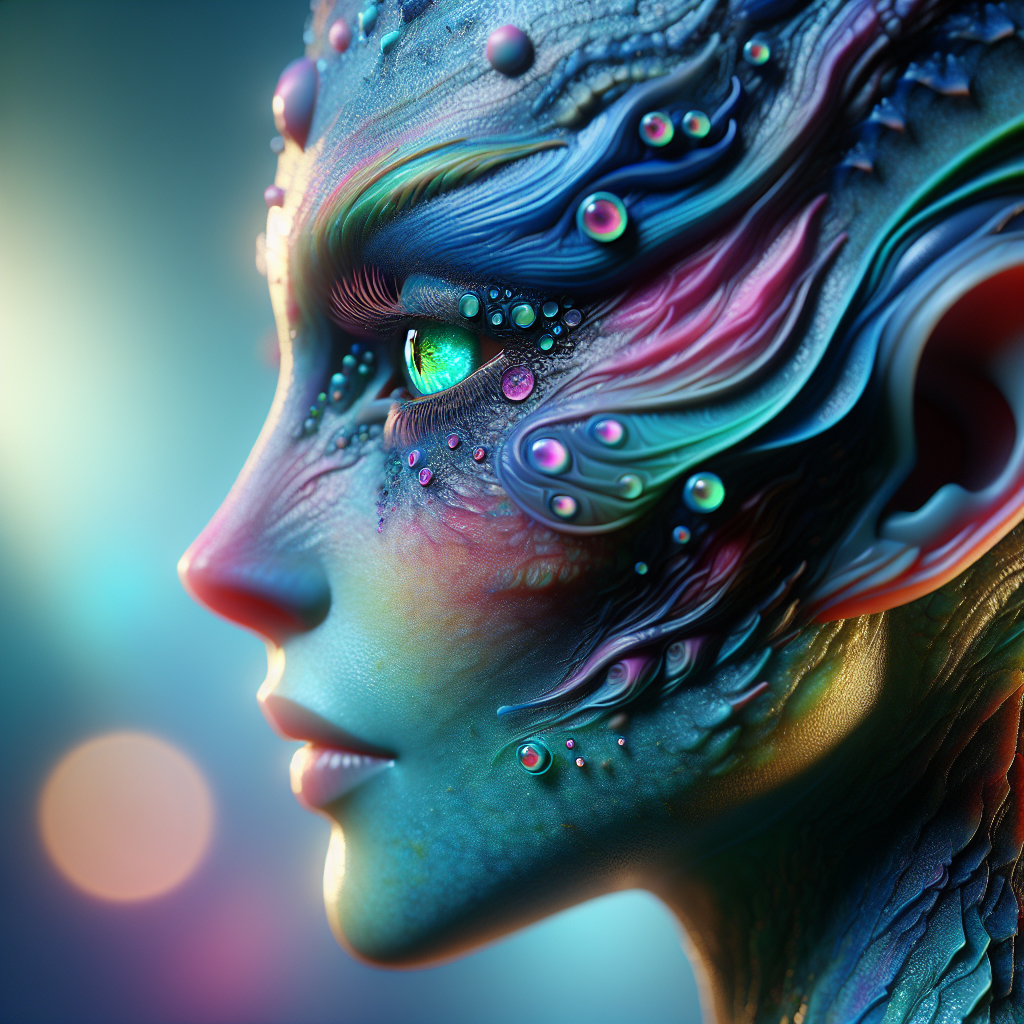
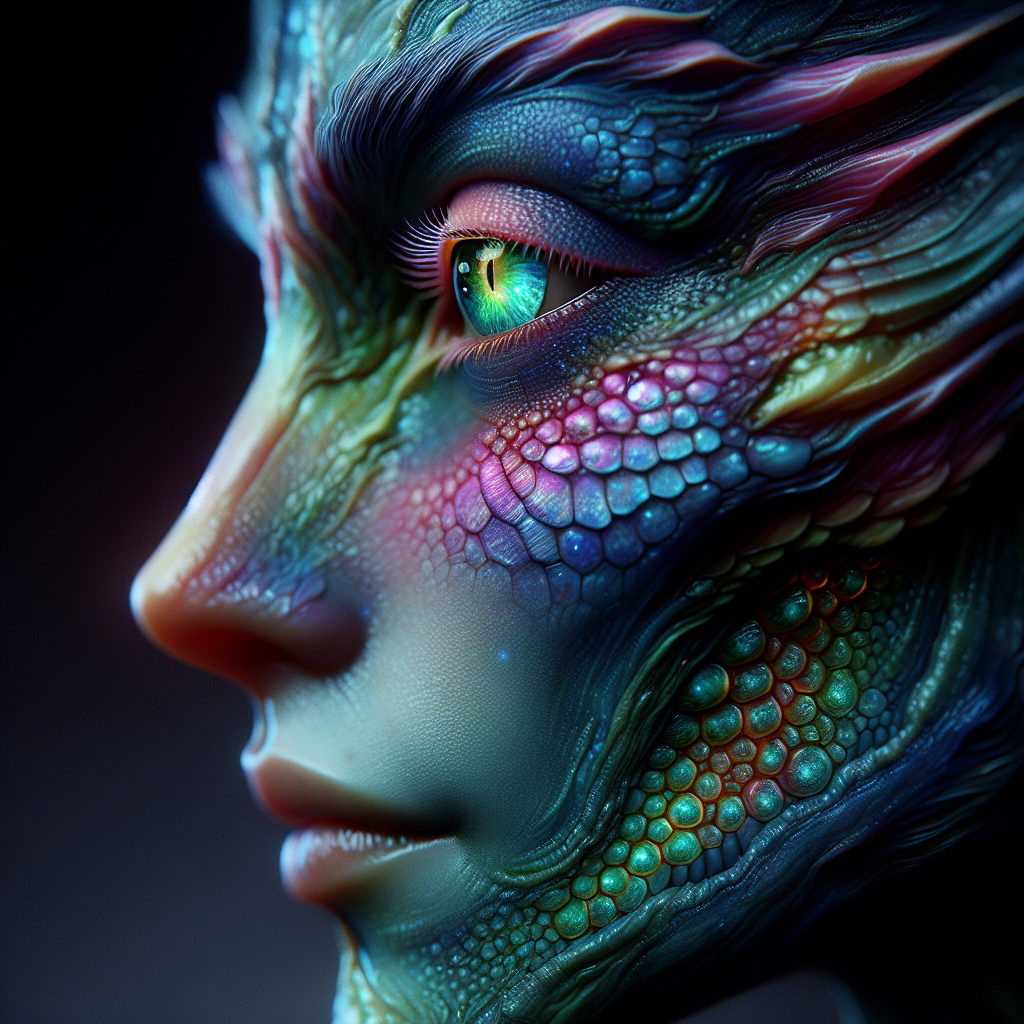
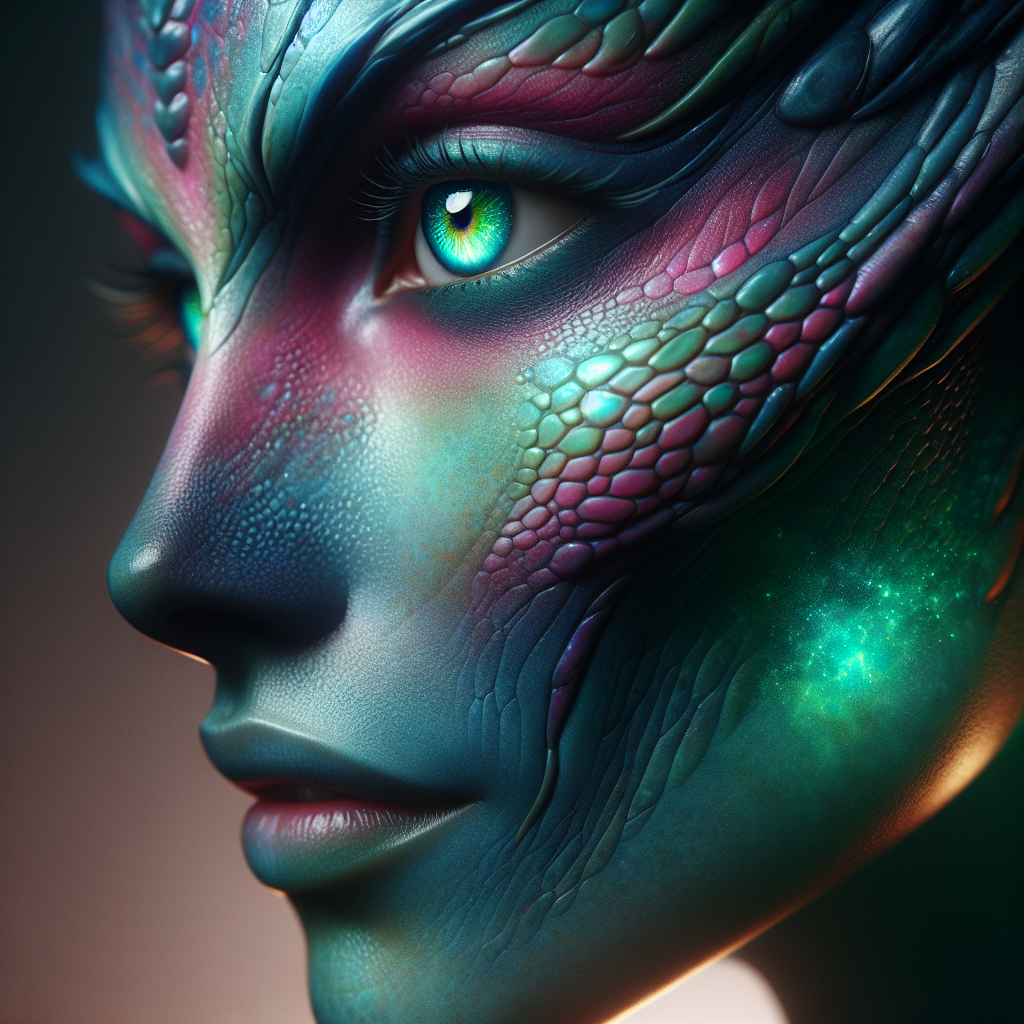
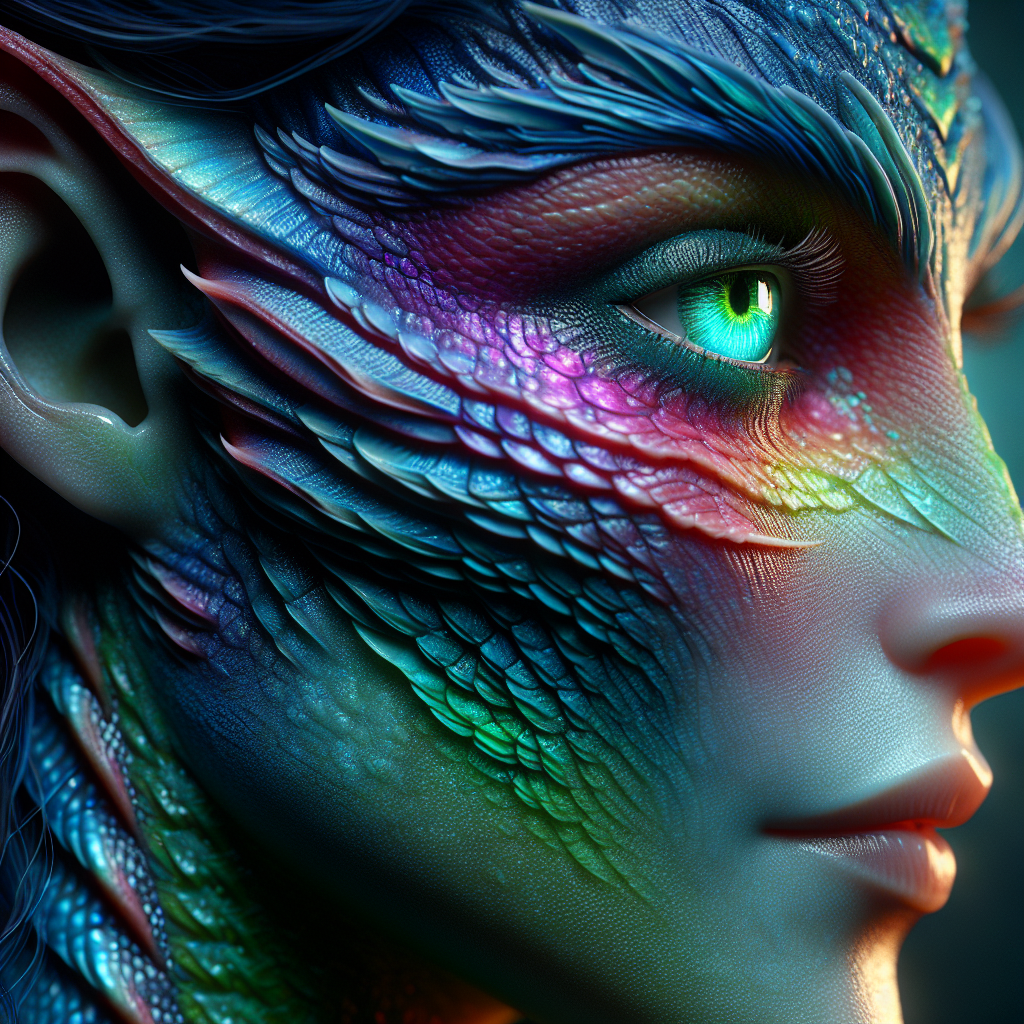
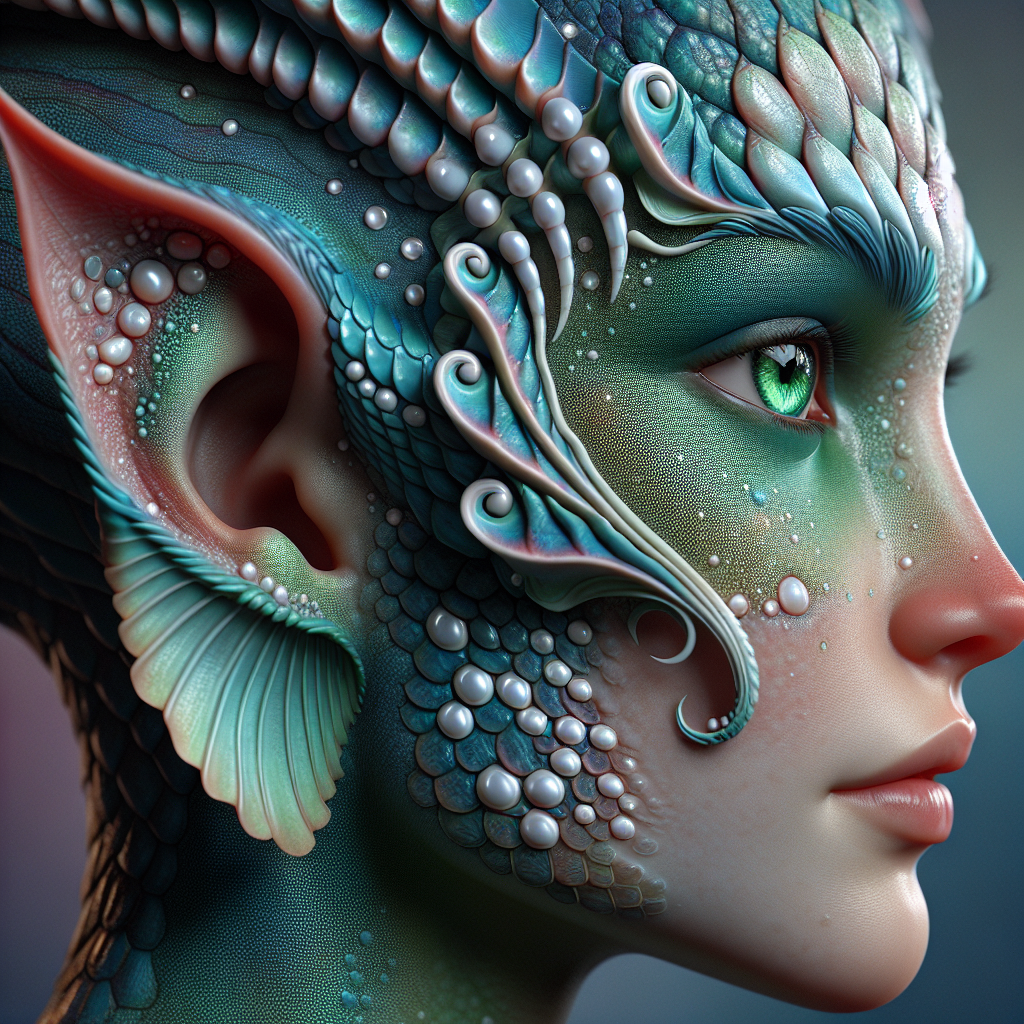
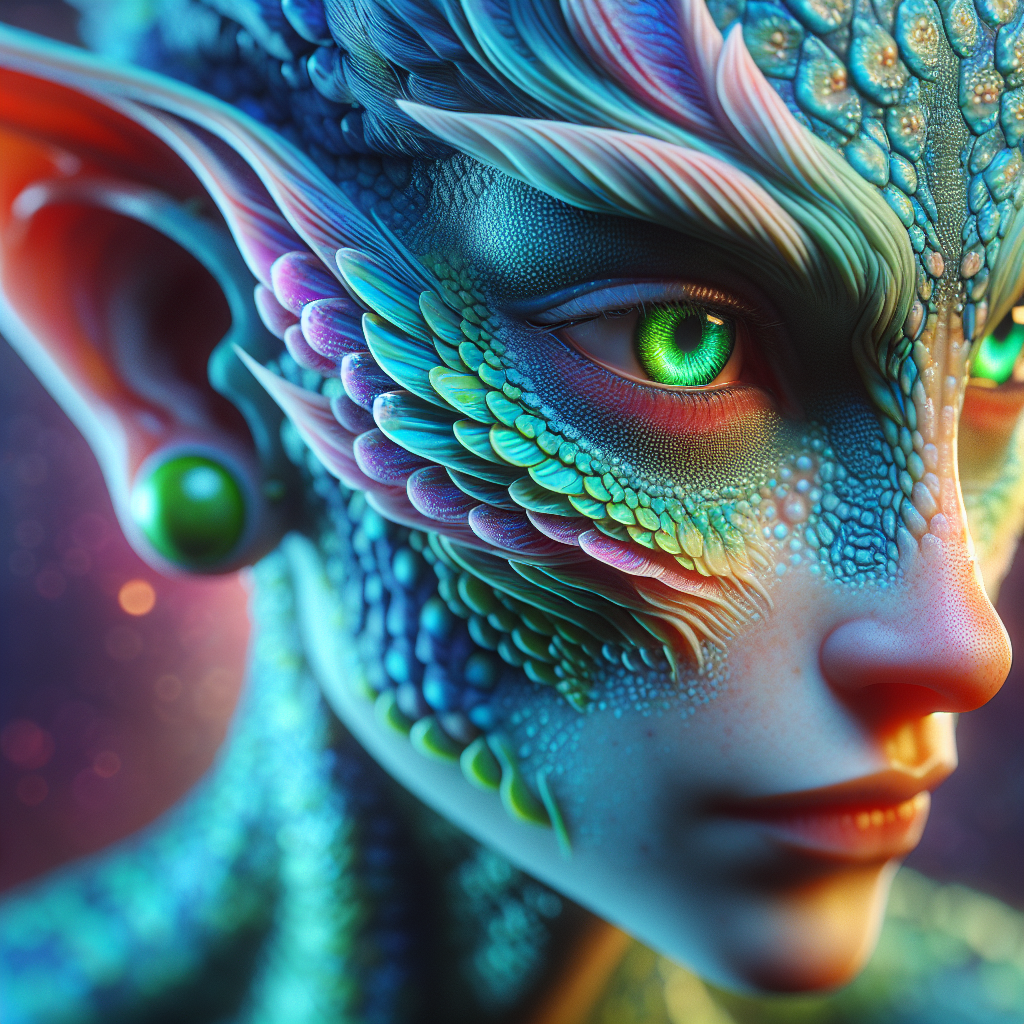
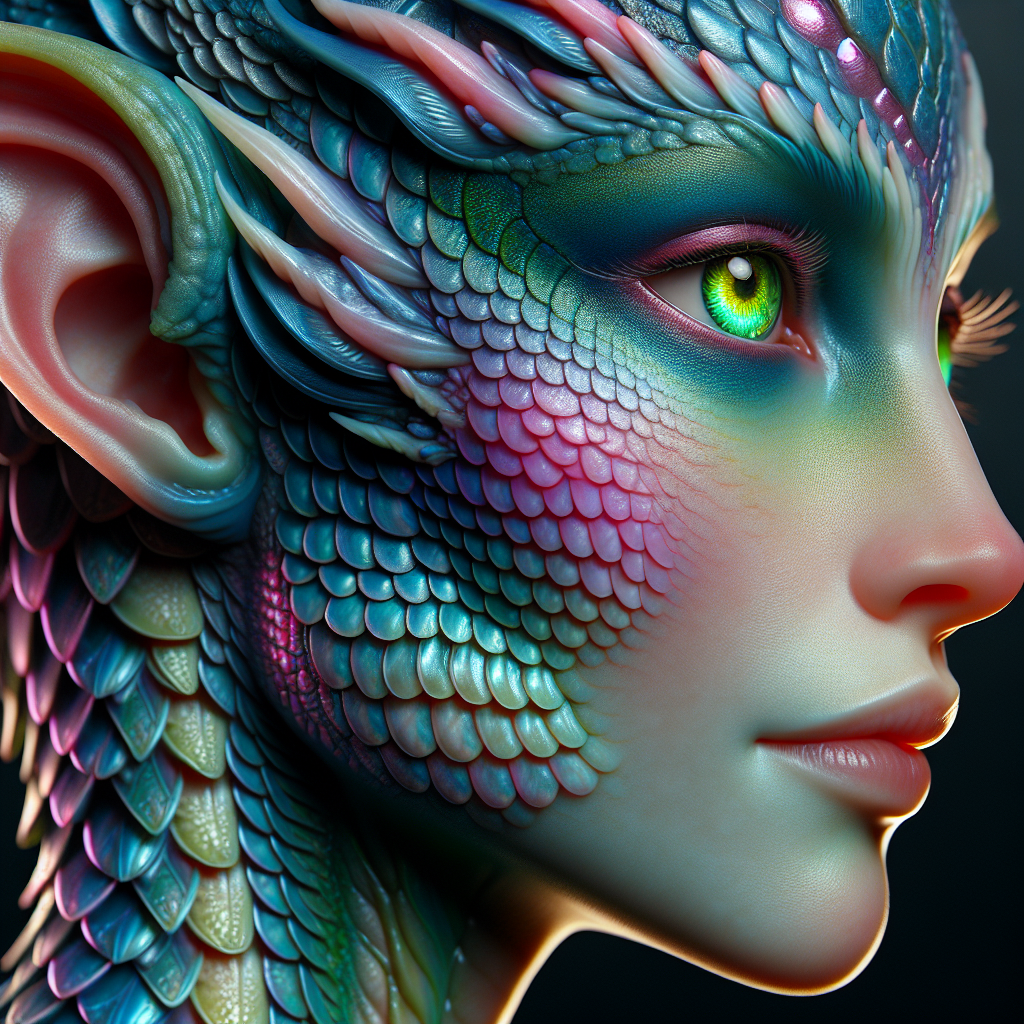
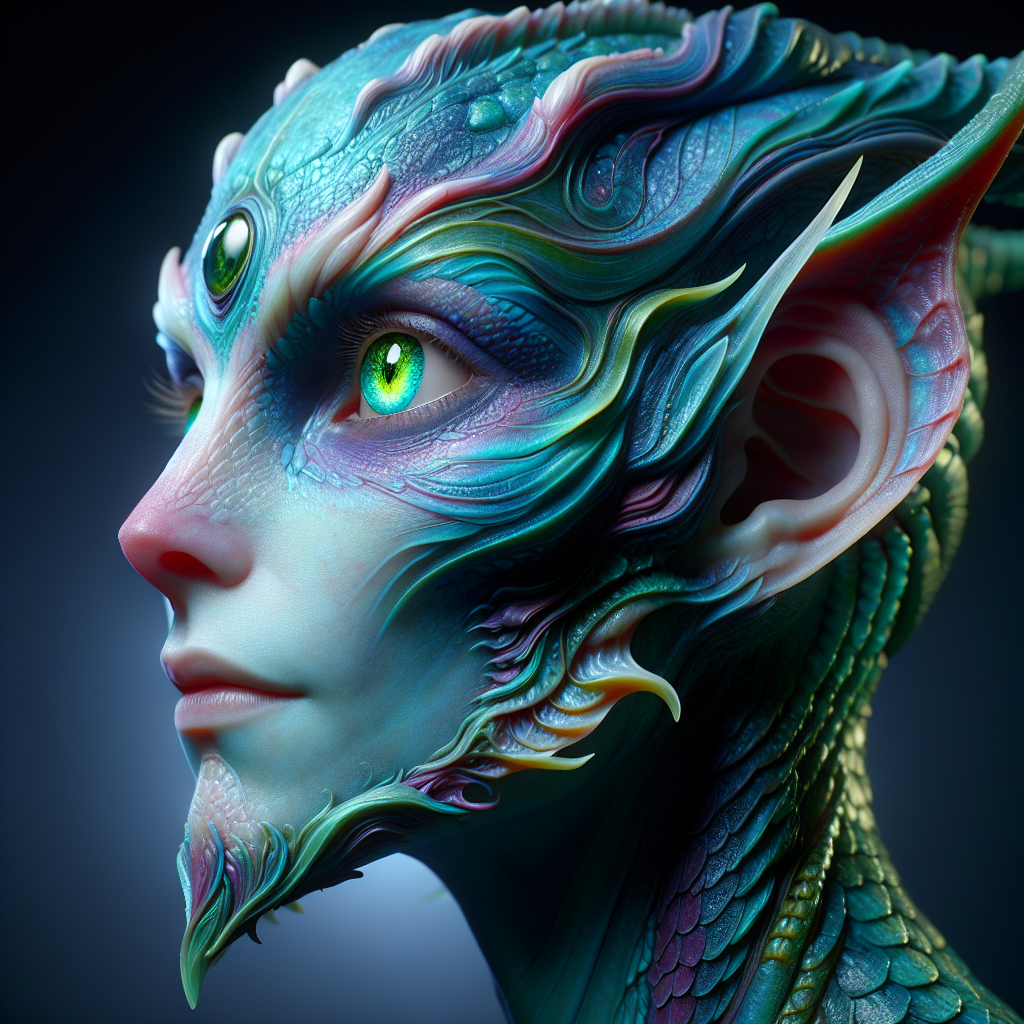
Conclusion
The synergy between GPT-4's descriptive capabilities and DALL-E 3's generative prowess showcases the remarkable potential of AI in the realm of digital art and image processing. Whether for artistic exploration, content creation, or research, this technology paves the way for innovative applications.
Remember, AI is a tool that thrives on creativity and experimentation. So, go ahead, tweak the code, try different images, and explore the endless possibilities!
To enhance your learning experience, it's highly recommended that you visit the accompanying visual guide provided in this blog post. This guide offers a detailed, step-by-step pictorial walkthrough that complements the written content, making it easier to understand and retain the information presented. Whether you're a visual learner or just looking for a more in-depth explanation, the illustrations in the guide will surely aid in your understanding of the topic.