Unlocking the Power of Voice: A Comprehensive Guide to Using OpenAI's Text-to-Speech API
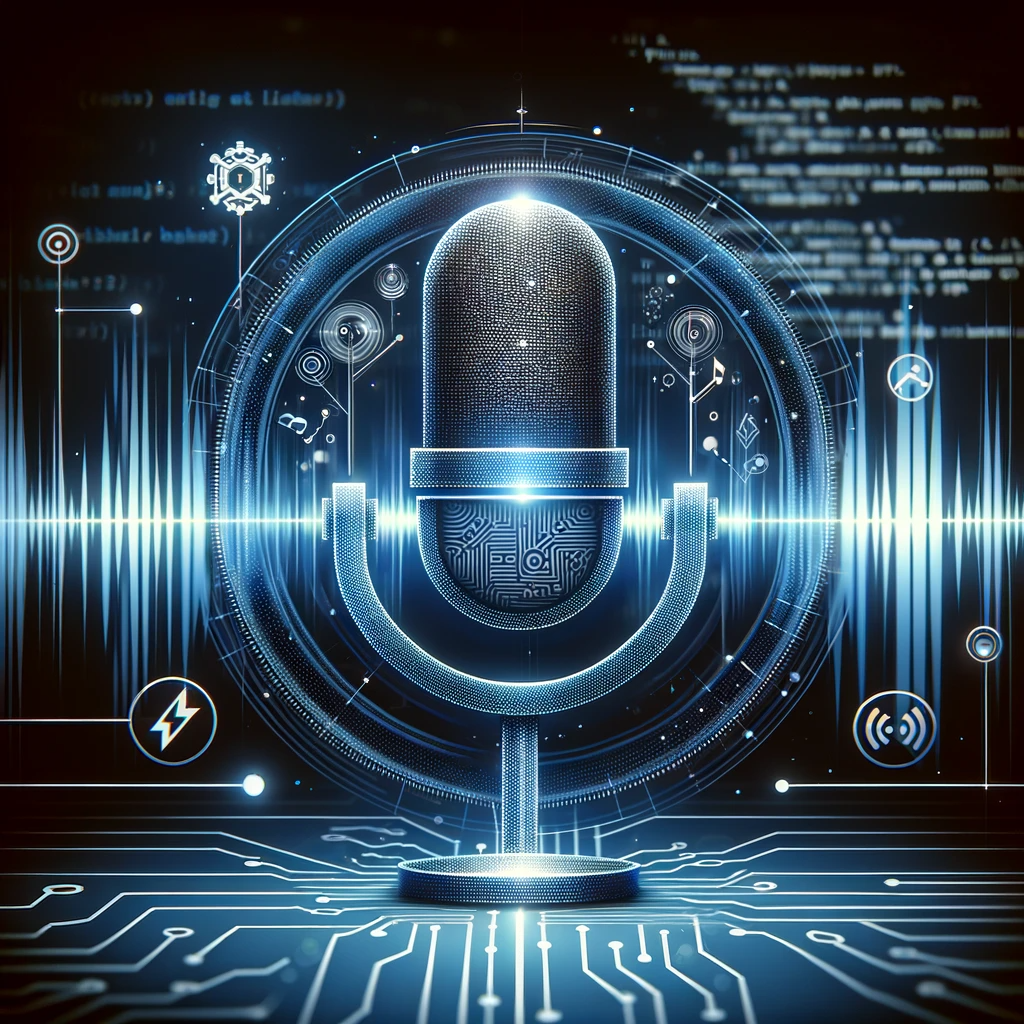
OpenAI's Text-to-Speech (TTS) API is a technology that transforms written text into spoken words, providing a natural-sounding voice output. The API offers two model variations:
- TTS-1: Optimized for real-time text-to-speech applications.
- TTS-1-HD: Focused on high-quality audio output.
This API comes with six prebuilt voices and can be used for narrating blog posts, producing multilingual audio, and offering real-time audio output. Users must disclose that the voice heard is AI-generated.
Getting Started with the TTS API:
- Prerequisites: An OpenAI account with funding, Python 3.7 or newer, and an Integrated Development Environment (IDE).
- Step 1: Generate an API Key: Log in to your OpenAI account, navigate to the API Keys section, and create a new secret key.
- Step 2: Create a Virtual Environment: This isolates your Python project and its dependencies.
from pathlib import Path
from openai import OpenAI
client = OpenAI()
speech_file_path = Path(__file__).parent / "speech.mp3"
response = client.audio.speech.create(
model="tts-1",
voice="alloy",
input="Today is a wonderful day to build something people love!"
)
response.stream_to_file(speech_file_path)
Bad Practice: Directly adding the key to the OpenAI object.
Good Practice: Using dotenv for key management.
pip install python-dotenv
Use Environment Variables:
import os
from pathlib import Path
from openai import OpenAI
from dotenv import load_dotenv
load_dotenv()
SECRET_KEY = os.getenv("SECRET_KEY")
client = OpenAI(api_key=SECRET_KEY)
speech_file_path = Path(__file__).parent / "speech.mp3"
response = client.audio.speech.create(
model="tts-1",
voice="alloy",
input="Today is a wonderful day to build something people love!"
)
response.stream_to_file(speech_file_path)
Customizing Voice and Output:
You can choose from six voices (Alloy, Echo, Fable, Onyx, Nova, Shimmer) and various output formats (MP3, AAC, FLAC, Opus).
Real-World Applications:
Useful for narrating content, creating multilingual audio, enhancing real-time interactions in games or with virtual assistants.
API Limits and Pricing:
- 50 RPM for paid accounts.
- Max input: 4,096 characters.
- Pricing varies between standard and HD models.
In summary, OpenAI's TTS API is a versatile tool for text-to-speech conversion, offering customization and suitable for a range of applications, with clear usage limits and cost guidelines.