Javascript Text To Speech: A Guide To Converting TTS With Javascript
Want to add a unique feature to your website? Learn how to integrate Javascript text-to-speech capabilities with this helpful guide.
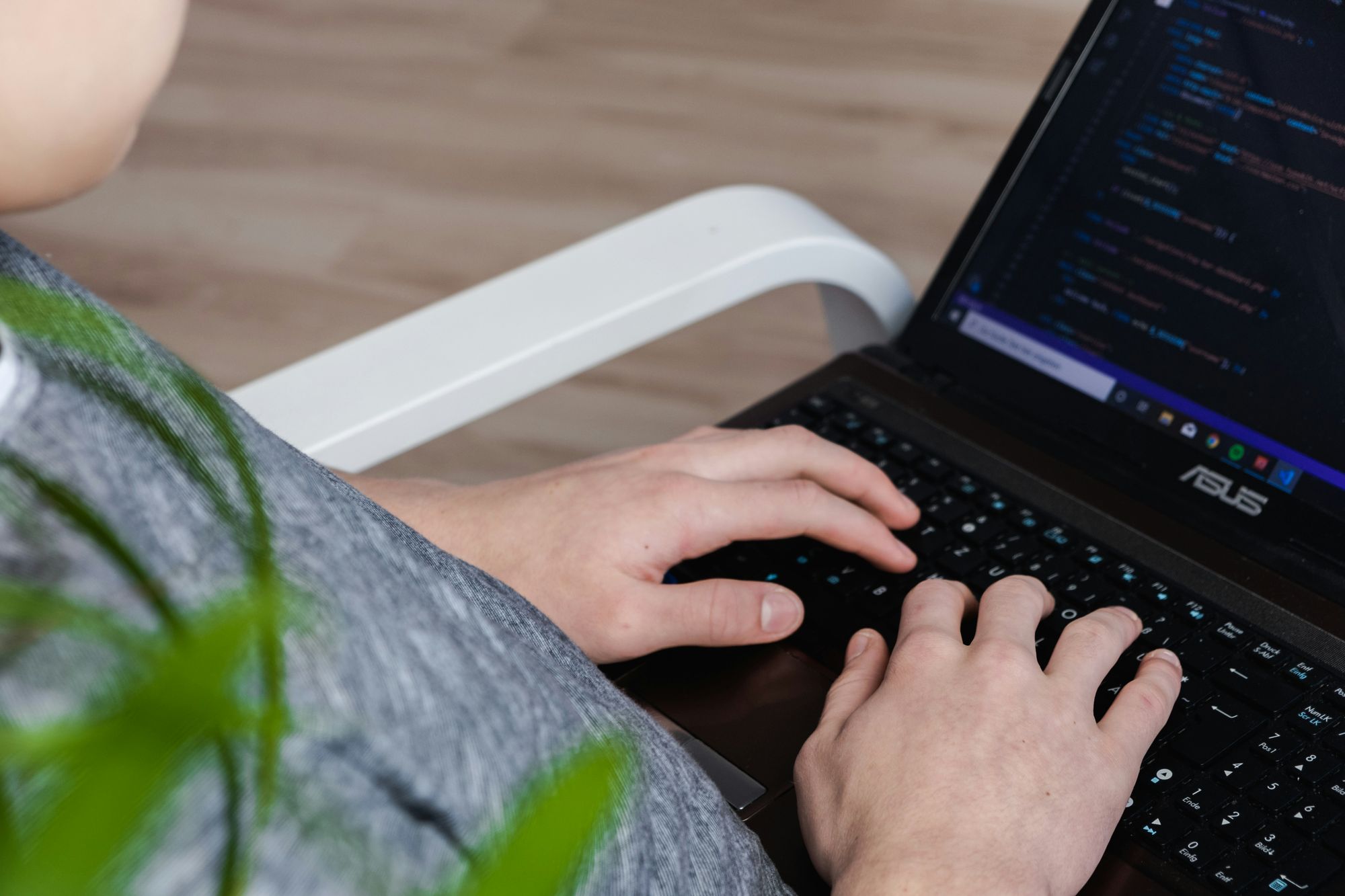
Javascript text to speech technology provides a unique digital experience by converting written content into spoken words. By leveraging Javascript text to speech, developers gain access to an array of features that can enhance user interaction and engagement. When applying text to speech technology, developers can implement accessibility features like screen readers, voice commands, and more. Through this technology, developers can bring an innovative and interactive dimension to their digital applications. Embrace the possibilities of Javascript text to speech and explore the endless potential it holds for your projects.
Table of Contents
- Introduction To JavaScript Text To Speech (TTS)
- Understanding JavaScript TTS APIs
- Getting Started With JavaScript Text To Speech
- Customizing The Speech To Your Preferences
- Best Practices For JavaScript TTS Development
- Try Unreal Speech for Free Today — Affordably and Scalably Convert Text into Natural-Sounding Speech with Our Text-to-Speech API
Introduction To JavaScript Text To Speech (TTS)
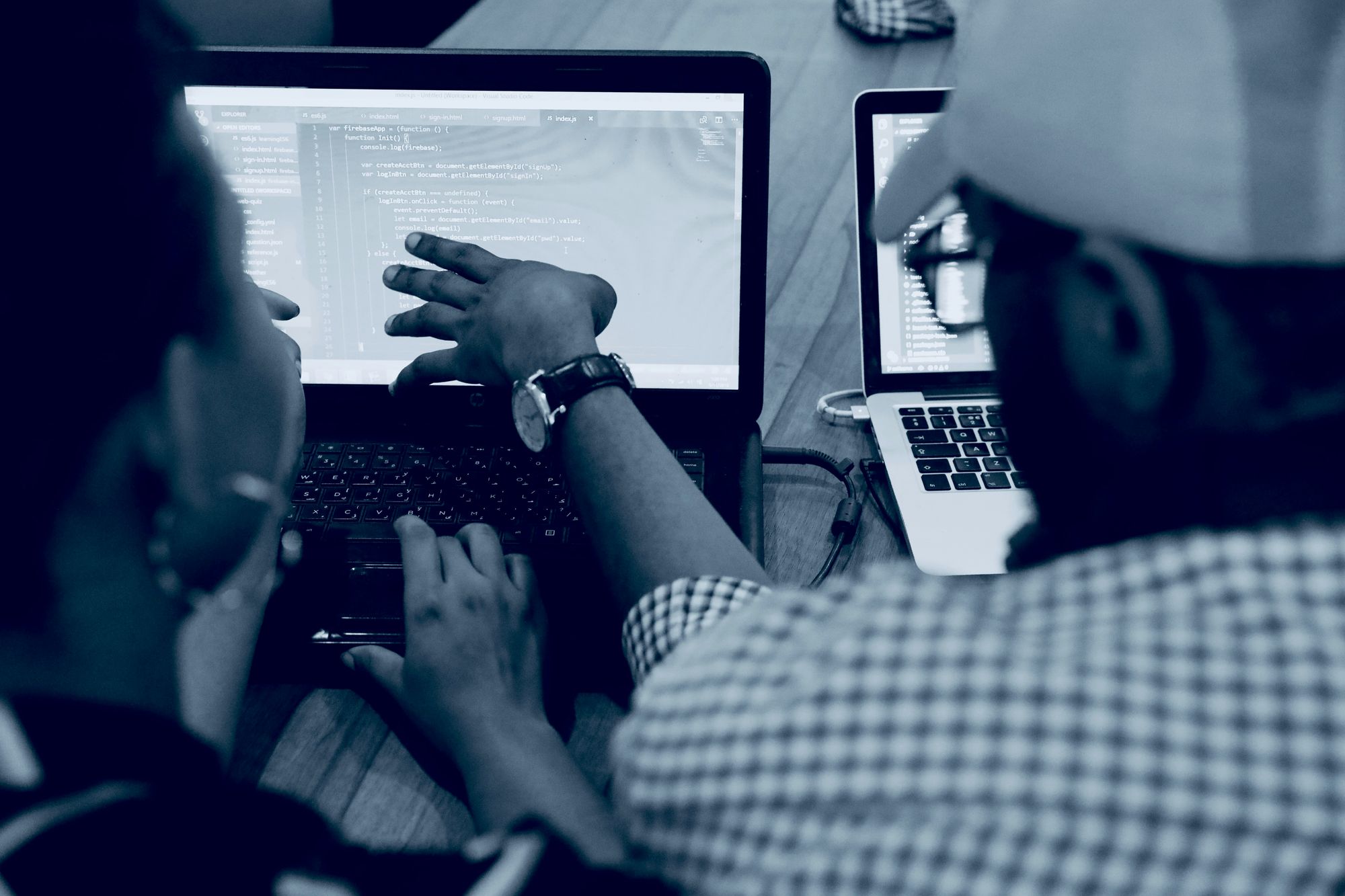
Text-to-Speech (TTS) technology enables computers to convert written text into spoken words. In the context of JavaScript, TTS allows developers to integrate speech synthesis capabilities directly into web applications. With TTS, users can interact with websites and applications using voice commands and receive audible feedback.
Importance of TTS in Web Development
TTS plays a crucial role in enhancing the accessibility and usability of web content. It enables users with visual impairments or reading difficulties to access online information more effectively by listening to the content instead of reading it.
TTS can provide a more engaging and interactive user experience, especially in applications that require hands-free operation or communication with users in diverse environments. Integrating TTS into web development projects can improve inclusivity, usability, and overall user satisfaction.
Cutting-Edge and Cost-Effective Text-to-Speech Solution
Unreal Speech offers a low-cost, highly scalable text-to-speech API with natural-sounding AI voices, which is the cheapest and most high-quality solution in the market. Unreal Speech can cut your text-to-speech costs by up to 90%. Get human-like AI voices with their super-fast, low-latency API, with the option for per-word timestamps. The simple, easy-to-use API allows you to give your LLM a voice with ease and offer this functionality at scale.
If you are looking for cheap, scalable, realistic TTS to incorporate into your products, try our text-to-speech API for free today. Convert text into natural-sounding speech at an affordable and scalable price.
Understanding JavaScript TTS APIs
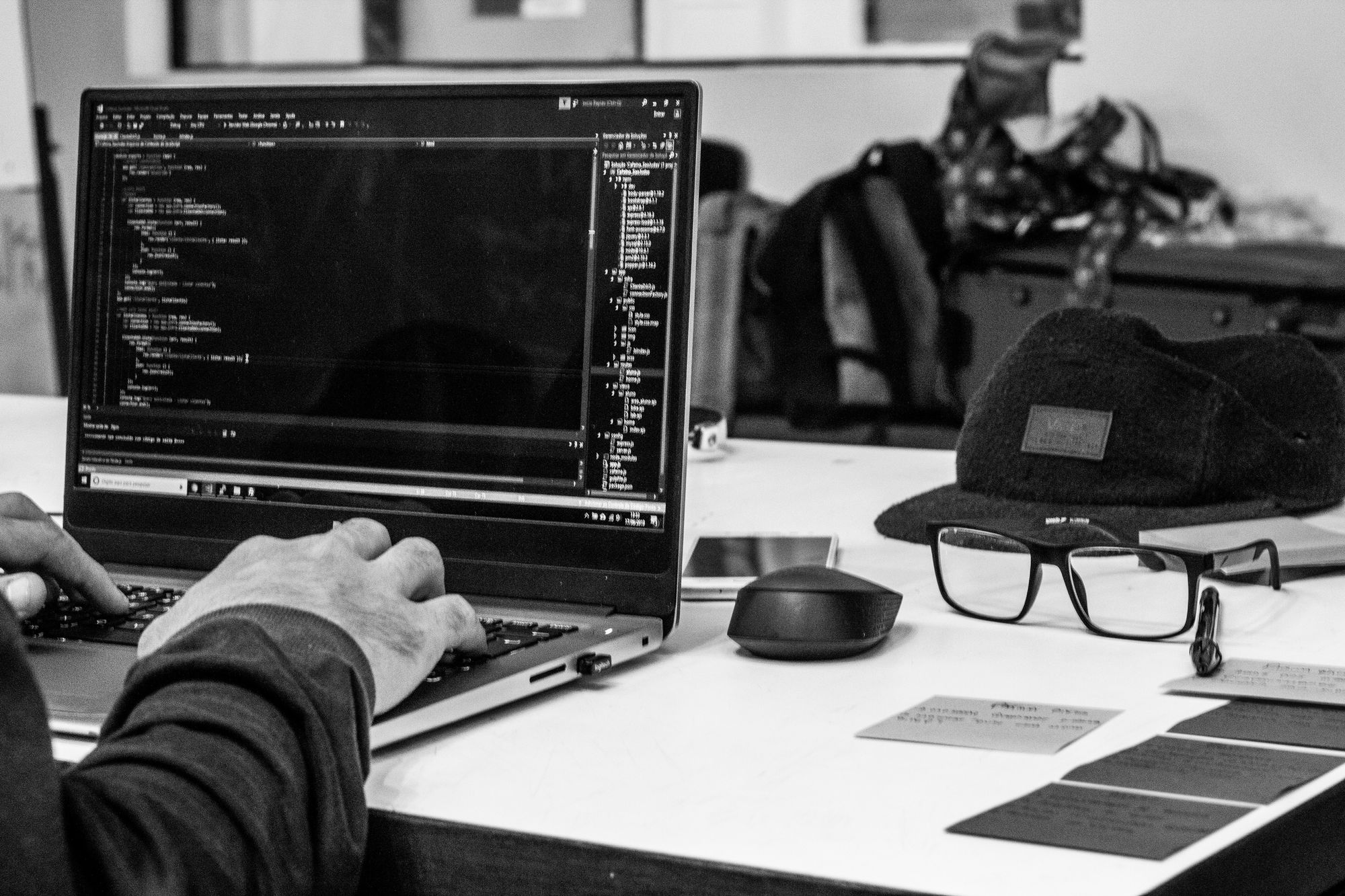
Web Speech API Overview
The Web Speech API provides a straightforward way to incorporate text-to-speech functionality into web applications using JavaScript. With just a few lines of code, developers can enable their browsers to convert text into speech. This API opens up a plethora of opportunities for enhancing user experiences and accessibility on the web.
TTS Functionality with Web Speech API
The Web Speech API offers developers various methods and functions to synthesize speech from plain text. Developers can further control speech synthesis parameters like voice selection and rate and handle related events effectively. While the Web Speech API's functionality largely depends on the voices available in the browser or the user's operating system, it provides a quick and cost-effective solution for integrating TTS capabilities into web applications.
Browser Compatibility and Support
Although the Web Speech API is supported by many modern web browsers such as Chrome, Firefox, and Safari, developers should be mindful of discrepancies in implementation across different platforms. Ensuring consistent behavior and fallback options is crucial for a seamless user experience. Testing browser compatibility and having fallback options ready can help maintain accessibility and functionality across various devices and platforms.
Affordable and Scalable Text-to-Speech Solution
If you are looking for cheap, scalable, realistic TTS to incorporate into your products, try our text-to-speech API for free today. Convert text into natural-sounding speech at an affordable and scalable price.
Getting Started With JavaScript Text To Speech
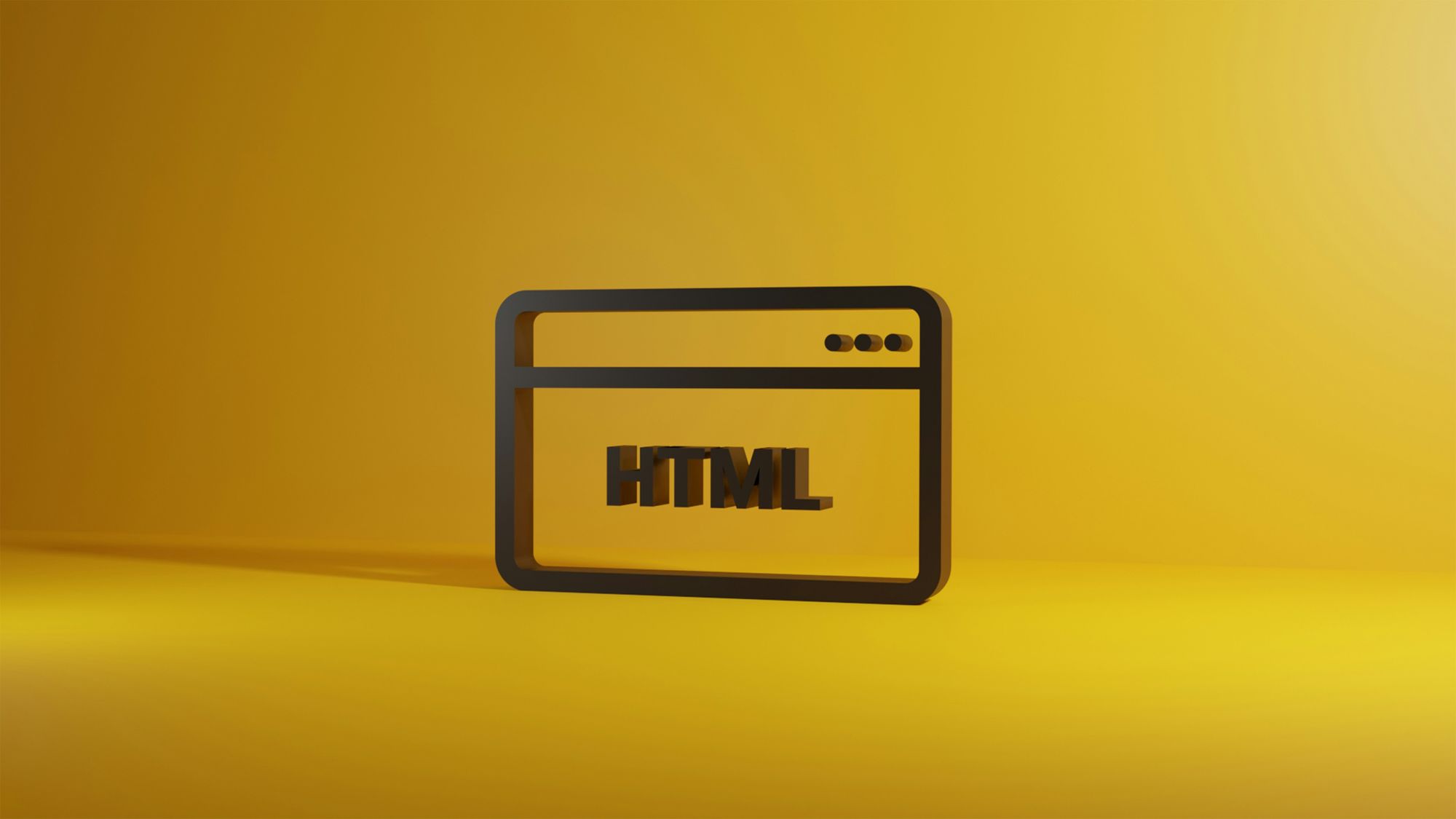
To utilize the Web Speech API for text-to-speech functionality in your JavaScript project, you need to include the necessary scripts in your HTML file. This typically involves referencing the SpeechSynthesis interface provided by the Web Speech API.
The speechSynthesis Interface
Begin by creating an HTML file and linking your JavaScript file using the script src tag. In your JavaScript file, initialize the speech synthesis object and set up an event listener for when the voices are ready.
```javascript
const synth = window.speechSynthesis;
// Wait for voices to be loaded
synth.onvoiceschanged = () => {
const voices = synth.getVoices();
// Do something with the available voices
};
```
Once the voices are loaded, you can access them using the synth.getVoices() method. This will return a list of available voices that you can use for speech synthesis. You can loop through the voices using forEach and display them in your HTML.
```javascript
const voiceSelect = document.getElementById(‘voice-select’);
voices.forEach((voice) => {
const option = document.createElement(‘option’);
option.textContent = ${voice.name} (${voice.lang});
option.setAttribute(‘value’, voice.lang);
voiceSelect.appendChild(option);
});
```
Next, you can create a function to synthesize speech from the selected voice. This function takes the text input from a textarea element and uses the selected voice to generate speech.
```javascript
const speak = () => {
const text = document.getElementById(‘text-input’).value;
const voice = voices[voiceSelect.selectedIndex];
const utterance = new SpeechSynthesisUtterance(text);
utterance.voice = voice;
synth.speak(utterance);
};
```
Add an event listener to the button or form submit to trigger the speak function.
```javascript
const button = document.getElementById(‘speak-button’);
button.addEventListener(‘click’, speak);
/
```
With these few lines of code, you can convert text to speech in real-time.
If you are looking for cheap, scalable, realistic TTS to incorporate into your products, try our text-to-speech API for free today. Convert text into natural-sounding speech at an affordable and scalable price.
Customizing The Speech To Your Preferences
To customize the speech rate, pitch, and volume with the Javascript text to speech, you can set properties on the SpeechSynthesisUtterance object. For the rate, a decimal value between 0.1 (slowest) and 10 (fastest) can be set, with 1 being the default value.
The pitch, on the other hand, is a decimal value between 0 (lowest) and 2 (highest; almost audible only for felines), with 1 being the default value. For a decent speech quality, you can customize these values with an example shown below:
- utterance.rate = 0.8;
- utterance.pitch = 1;
- utterance.volume = 1;
Best Practices For JavaScript TTS Development
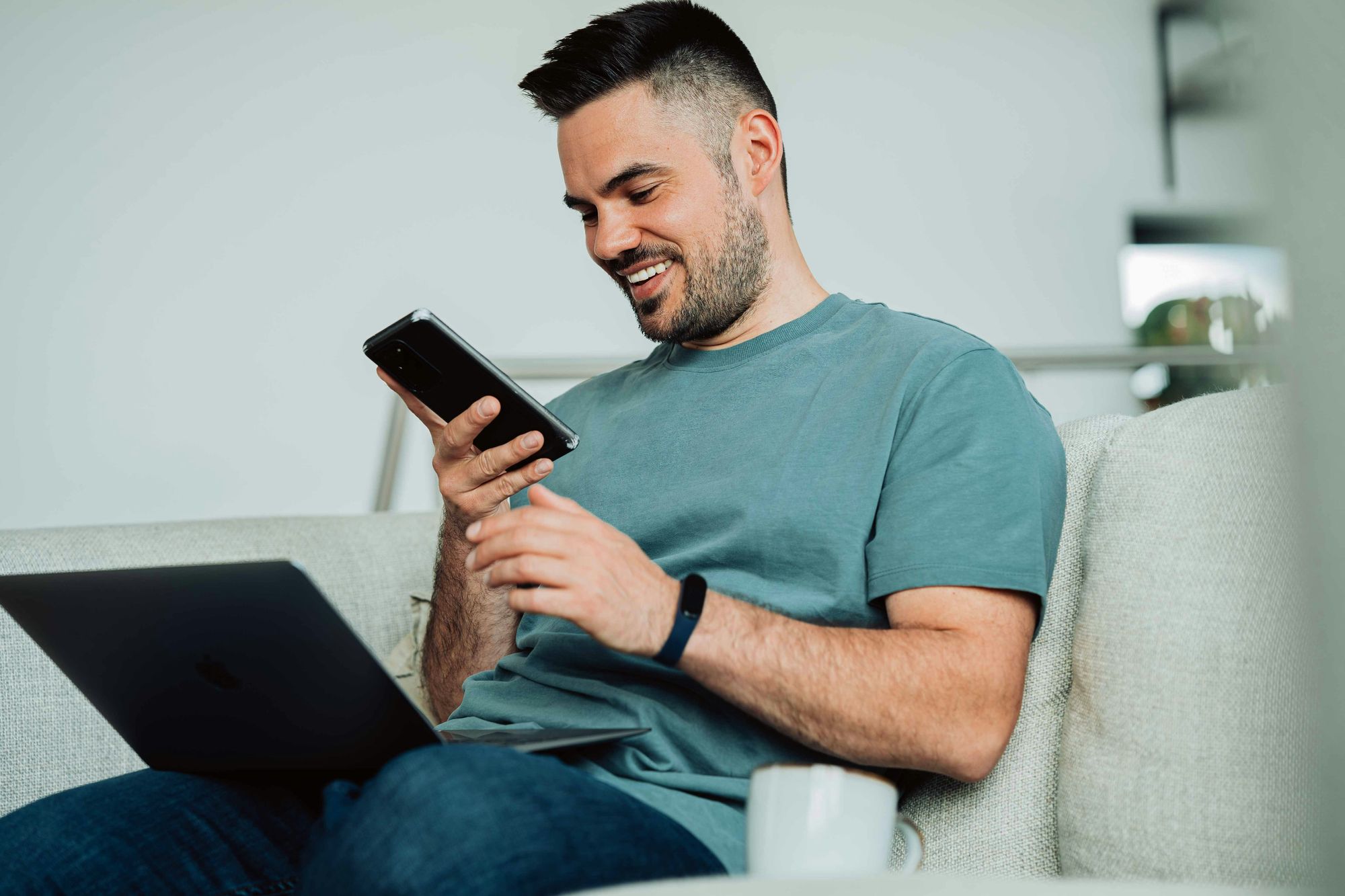
Efficient Algorithms for Text-to-Speech Processing
When developing a JavaScript TTS system, I always make sure to implement efficient algorithms that can handle large volumes of text quickly and accurately. By using efficient algorithms, I can drastically reduce latency and resource consumption, resulting in a smoother and more responsive user experience.
Caching Frequently Used Data for Improved Performance
Caching frequently accessed or processed text data is vital for optimizing the performance of my JavaScript TTS applications. By storing commonly used text data in a cache, I can avoid repetitive computations during speech synthesis, thereby reducing the overhead and enhancing the overall efficiency of the system.
Testing Across Multiple Browsers for Compatibility
One of the key best practices I follow for JavaScript TTS development is to ensure that my implementation works seamlessly across various web browsers and versions. By validating my JavaScript TTS code across multiple browsers, I can identify and address any compatibility issues, guaranteeing a consistent user experience across different platforms.
Handling Browser-Specific Behavior with Fallbacks
To account for differences in browser behavior and feature support, I always incorporate fallback mechanisms or polyfills in my JavaScript TTS applications. By doing so, I can ensure that my TTS system functions correctly even in browsers that lack full support for the Web Speech API, thereby enhancing the overall reliability and robustness of the application.
Comprehensive Testing for Enhanced TTS Functionality
Thorough testing is a crucial aspect of developing a successful JavaScript TTS system. I test my TTS functionality across a wide range of scenarios, including varying text inputs, speech settings, and user interactions. Through comprehensive testing, I can identify and address any potential issues or limitations, ensuring that my JavaScript TTS implementation delivers a seamless and flawless user experience.
Try Unreal Speech for Free Today — Affordably and Scalably Convert Text into Natural-Sounding Speech with Our Text-to-Speech API
Unreal Speech is a game-changer in the world of text-to-speech API solutions. By using Unreal Speech, you can experience a drastic reduction in the costs associated with text-to-speech, potentially up to a whopping 90%. A unique aspect of Unreal Speech is its capability to provide you with a highly scalable text-to-speech API.
Thus, whether your project is small or large-scale, Unreal Speech has your back. And the cherry on top? The quality of the speech generated by this tool is top-notch, with AI voices that sound very natural. These voices are so close to human-like that you might have to remind yourself that it's actually a machine speaking!
Precision and Ease of Use with Per-Word Timestamps
Unreal Speech also boasts a feature that is incredibly beneficial for many projects: per-word timestamps. Developers like me often need such precision when it comes to generating speech from text. Thus, having per-word timestamps as a feature is not only convenient but also a necessity.
Speaking of convenience, Unreal Speech is designed with a simple API that is easy to use. In Javascript Text-to-Speech, I can vouch for the fact that a simple interface is a blessing when working on projects that involve generating speech.
Unreal Speech's Fast Operation
Another amazing feature of Unreal Speech is the speed at which it operates. With super low latency, you can expect the tool to work fast, getting you your results in no time. This is a huge plus point, particularly when working on time-sensitive projects. Therefore, if you’re looking for an affordable and scalable text-to-speech solution that offers high-quality output and is easy to integrate into your products, Unreal Speech is the way to go.
Give it a try today!